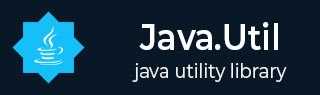
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Timer purge() Method
Description
The Java Timer purge() method is used to remove all cancelled tasks from this timer's task queue.
Declaration
Following is the declaration for java.util.Timer.purge() method.
public int purge()
Parameters
NA
Return Value
The method call returns the number of tasks removed from the queue.
Exception
NA
Purging a Timer Scheduled at Fixed Rate Example
The following example shows the usage of Java Timer purge() method to purge the timer operations currently scheduled. We've created a timer object using a CustomTimerTask object. CustomTimerTask is custom class extending TimerTask class and implements the run() method which will execute at scheduled time. Then we created a timer object and scheduled a task using scheduleAtFixedRate() to execute at every 100 milliseconds starting from now. Then using cancel() method, the timer operation is terminated and using purge() method, cancelled task is removed from the queue.
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer(); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); // terminating the timer System.out.println("cancelling timer"); timer.cancel(); System.out.println("purge value :"+timer.purge()); } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
Output
Let us compile and run the above program, this will produce the following result.
cancelling timer purge value :0 working on
Purging a Timer Scheduled at Fixed Rate and Date Example
The following example shows the usage of Java Timer purge() method to purge the timer operations currently scheduled. We've created a timer object using a CustomTimerTask object. CustomTimerTask is custom class extending TimerTask class and implements the run() method which will execute at scheduled time. Then we created a timer object as a daemon thread and scheduled a task using scheduleAtFixedRate() to execute at every 100 milliseconds starting from now. Then using cancel() method, the timer operation is terminated and using purge() method, cancelled task is removed from the queue.
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer(true); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); // terminating the timer System.out.println("cancelling timer"); timer.cancel(); System.out.println("purge value :"+timer.purge()); } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
Output
Let us compile and run the above program, this will produce the following result.
cancelling timer purge value :0 working on
Purging a Timer Scheduled at Fixed Date and Initial Delay Example
The following example shows the usage of Java Timer purge() method to purge the timer operations currently scheduled. We've created a timer object using a CustomTimerTask object. CustomTimerTask is custom class extending TimerTask class and implements the run() method which will execute at scheduled time. Then we created a timer object as a daemon thread, with a given name and scheduled a task using scheduleAtFixedRate() to execute at every 100 milliseconds starting from now. Then using cancel() method, the timer operation is terminated and using purge() method, cancelled task is removed from the queue.
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer("test",true); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); // terminating the timer System.out.println("cancelling timer"); timer.cancel(); System.out.println("purge value :"+timer.purge()); } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
Output
Let us compile and run the above program, this will produce the following result.
cancelling timer purge value :0 working on