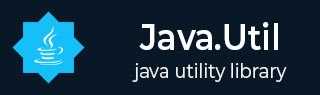
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java SimpleTimeZone clone() Method
Description
The Java SimpleTimeZone clone() method is used to return a clone of this SimpleTimeZone instance.
Declaration
Following is the declaration for java.util.SimpleTimeZone.clone() method.
public Object clone()
Parameters
NA
Return Value
The method call returns a clone of this instance.
Exception
NA
Cloning a SimpleTimeZone Instane of India Timezone Example
The following example shows the usage of Java SimpleTimeZone clone() method to create a clone of SimpleTimeZone object. We've created a SimpleTimeZone using India then printed it. Then we've created the clone of this object using clone() method and printed it as well.
package com.tutorialspoint; import java.util.SimpleTimeZone; public class SimpleTimeZoneDemo { public static void main( String args[] ) { // create simple time zone object SimpleTimeZone stobj = new SimpleTimeZone(720,"India"); System.out.println("Original obj: " + stobj); // clone the object Object cloneObj = stobj.clone(); System.out.println("Clone obj: " + cloneObj); } }
Output
Let us compile and run the above program, this will produce the following result.
Original obj: java.util.SimpleTimeZone[id=India,offset=720,dstSavings=3600000,useDaylight=false,startYear=0,startMode=0,startMonth=0,startDay=0,startDayOfWeek=0,startTime=0,startTimeMode=0,endMode=0,endMonth=0,endDay=0,endDayOfWeek=0,endTime=0,endTimeMode=0] Clone obj: java.util.SimpleTimeZone[id=India,offset=720,dstSavings=3600000,useDaylight=false,startYear=0,startMode=0,startMonth=0,startDay=0,startDayOfWeek=0,startTime=0,startTimeMode=0,endMode=0,endMonth=0,endDay=0,endDayOfWeek=0,endTime=0,endTimeMode=0]
Cloning a SimpleTimeZone Instane of America Timezone Example
The following example shows the usage of Java SimpleTimeZone clone() method to create a clone of SimpleTimeZone object. We've created a SimpleTimeZone using America/Los_Angeles then printed it. Then we've created the clone of this object using clone() method and printed it as well.
package com.tutorialspoint; import java.util.SimpleTimeZone; public class SimpleTimeZoneDemo { public static void main( String args[] ) { // create simple time zone object SimpleTimeZone stobj = new SimpleTimeZone(720,"America/Los_Angeles"); System.out.println("Original obj: " + stobj); // clone the object Object cloneObj = stobj.clone(); System.out.println("Clone obj: " + cloneObj); } }
Output
Let us compile and run the above program, this will produce the following result.
Original obj: java.util.SimpleTimeZone[id=America/Los_Angeles,offset=720,dstSavings=3600000,useDaylight=false,startYear=0,startMode=0,startMonth=0,startDay=0,startDayOfWeek=0,startTime=0,startTimeMode=0,endMode=0,endMonth=0,endDay=0,endDayOfWeek=0,endTime=0,endTimeMode=0] Clone obj: java.util.SimpleTimeZone[id=America/Los_Angeles,offset=720,dstSavings=3600000,useDaylight=false,startYear=0,startMode=0,startMonth=0,startDay=0,startDayOfWeek=0,startTime=0,startTimeMode=0,endMode=0,endMonth=0,endDay=0,endDayOfWeek=0,endTime=0,endTimeMode=0]
Cloning a SimpleTimeZone Instane of Europe Timezone Example
The following example shows the usage of Java SimpleTimeZone clone() method to create a clone of SimpleTimeZone object. We've created a SimpleTimeZone using Europe/Paris then printed it. Then we've created the clone of this object using clone() method and printed it as well.
package com.tutorialspoint; import java.util.SimpleTimeZone; public class SimpleTimeZoneDemo { public static void main( String args[] ) { // create simple time zone object SimpleTimeZone stobj = new SimpleTimeZone(720,"Europe/Paris"); System.out.println("Original obj: " + stobj); // clone the object Object cloneObj = stobj.clone(); System.out.println("Clone obj: " + cloneObj); } }
Output
Let us compile and run the above program, this will produce the following result.
Original obj: java.util.SimpleTimeZone[id=Europe/Paris,offset=720,dstSavings=3600000,useDaylight=false,startYear=0,startMode=0,startMonth=0,startDay=0,startDayOfWeek=0,startTime=0,startTimeMode=0,endMode=0,endMonth=0,endDay=0,endDayOfWeek=0,endTime=0,endTimeMode=0] Clone obj: java.util.SimpleTimeZone[id=Europe/Paris,offset=720,dstSavings=3600000,useDaylight=false,startYear=0,startMode=0,startMonth=0,startDay=0,startDayOfWeek=0,startTime=0,startTimeMode=0,endMode=0,endMonth=0,endDay=0,endDayOfWeek=0,endTime=0,endTimeMode=0]
To Continue Learning Please Login