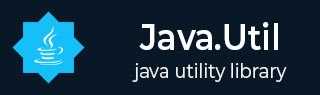
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Observable hasChanged() Method
Description
The Java Observable hasChanged() method returns if this object has changed.
Declaration
Following is the declaration for Java.util.Observable.hasChanged() method
public boolean hasChanged()
Parameters
NA
Return Value
NA
Exception
NA
Checking Observed of String Value for Change Example
The following example shows the usage of java.util.Observable.hasChanged() method. We've created an ObservedObject class by extending Observable class and then overridden its method setValue(). In main class, We've added the observers using addObserver() method. Then using hasChanged(), we've printed the updates happening to the value of ObservedObject.
package com.tutorialspoint; import java.util.Observable; import java.util.Observer; class ObservedObject extends Observable { private String watchedValue; public ObservedObject(String value) { watchedValue = value; } public void setValue(String value) { // if value has changed notify observers if(!watchedValue.equals(value)) { watchedValue = value; // mark as value changed setChanged(); } } } public class ObservableDemo implements Observer { public String name; public ObservableDemo(String name) { this.name = name; } public static void main(String[] args) { // create watched and watcher objects ObservedObject watched = new ObservedObject("Original Value"); // watcher object listens to object change ObservableDemo watcher = new ObservableDemo("Watcher"); // add observer to the watched object watched.addObserver(watcher); // trigger value change System.out.println("setValue method called..."); watched.setValue("New Value"); // check if value has changed if(watched.hasChanged()) System.out.println("Value changed"); else System.out.println("Value not changed"); } public void update(Observable obj, Object arg) { System.out.println("Update called"); } }
Output
Let us compile and run the above program, this will produce the following result −
setValue method called... Value changed
Checking Observed of Integer Value for Change Example
The following example shows the usage of java.util.Observable.hasChanged() method. We've created an ObservedObject class by extending Observable class and then overridden its method setValue(). In main class, We've added the observers using addObserver() method. Then using hasChanged(), we've printed the updates happening to the value of ObservedObject.
package com.tutorialspoint; import java.util.Observable; import java.util.Observer; class ObservedObject extends Observable { private Integer watchedValue; public ObservedObject(Integer value) { watchedValue = value; } public void setValue(Integer value) { // if value has changed notify observers if(!watchedValue.equals(value)) { watchedValue = value; // mark as value changed setChanged(); } } } public class ObservableDemo implements Observer { public String name; public ObservableDemo(String name) { this.name = name; } public static void main(String[] args) { // create watched and watcher objects ObservedObject watched = new ObservedObject(1); // watcher object listens to object change ObservableDemo watcher = new ObservableDemo("Watcher"); // add observer to the watched object watched.addObserver(watcher); // trigger value change System.out.println("setValue method called..."); watched.setValue(2); // check if value has changed if(watched.hasChanged()) System.out.println("Value changed"); else System.out.println("Value not changed"); } public void update(Observable obj, Object arg) { System.out.println("Update called"); } }
Output
Let us compile and run the above program, this will produce the following result −
setValue method called... Value changed
Checking Observed of Object Value for Change Example
The following example shows the usage of java.util.Observable.hasChanged() method. We've created an ObservedObject class by extending Observable class and then overridden its method setValue(). In main class, We've added the observers using addObserver() method. Then using hasChanged(), we've printed the updates happening to the value of ObservedObject.
package com.tutorialspoint; import java.util.Observable; import java.util.Observer; class ObservedObject extends Observable { private Student watchedValue; public ObservedObject(Student value) { watchedValue = value; } public void setValue(Student value) { // if value has changed notify observers if(!watchedValue.equals(value)) { watchedValue = value; // mark as value changed setChanged(); } } } public class ObservableDemo implements Observer { public String name; public ObservableDemo(String name) { this.name = name; } public static void main(String[] args) { // create watched and watcher objects ObservedObject watched = new ObservedObject(new Student(1, "Julie")); // watcher object listens to object change ObservableDemo watcher = new ObservableDemo("Watcher"); // add observer to the watched object watched.addObserver(watcher); // trigger value change System.out.println("setValue method called..."); watched.setValue(new Student(2, "Robert")); // check if value has changed if(watched.hasChanged()) System.out.println("Value changed"); else System.out.println("Value not changed"); } public void update(Observable obj, Object arg) { System.out.println("Update called"); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
setValue method called... Value changed
To Continue Learning Please Login