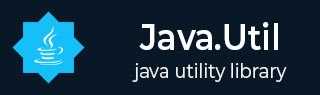
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java ListResourceBundle getContents() Method
Description
The Java ListResourceBundle getContents() method returns an array in which each item is a pair of objects in an Object array. The first element of each pair is the key, which must be a String, and the second element is the value associated with that key.
Declaration
Following is the declaration for java.util.ListResourceBundle.getContents() method
protected abstract Object[][] getContents()
Parameters
NA
Return Value
This method returns an array of an Object array representing a key-value pair.
Exception
NA
Defining Content of ListResourceBundle of String, Integer Pair Example
The following example shows the usage of Java ListResourceBundle getContents() method to add entries to the ListResourceBundle. We've created a ListResourceBundle object having contents an two dimensional array of String, Integer pairs. Then few entries are added by overriding getContents() method and then a value is retrieved using key and is printed.
package com.tutorialspoint; import java.util.Enumeration; import java.util.ListResourceBundle; // create a class that extends to ListResourceBundle class MyResources extends ListResourceBundle { // get contents must be implemented protected Object[][] getContents() { return new Object[][] { {"1", 1}, {"2", 2}, {"3", 3} }; } } public class ListResourceBundleDemo { public static void main(String[] args) { // create a new MyResources instance MyResources mr = new MyResources(); // print the value for key 1 System.out.println("" + mr.handleGetObject("1")); } }
Output
Let us compile and run the above program, this will produce the following result.
1 2 3
Defining Content of ListResourceBundle of String, String Pair Example
The following example shows the usage of Java ListResourceBundle getContents() method to add entries to the ListResourceBundle. We've created a ListResourceBundle object having contents an two dimensional array of String, String pairs. Then few entries are added by overriding getContents() method and then a value is retrieved using key and is printed.
package com.tutorialspoint; import java.util.Enumeration; import java.util.ListResourceBundle; // create a class that extends to ListResourceBundle class MyResources extends ListResourceBundle { // get contents must be implemented protected Object[][] getContents() { return new Object[][] { {"1", "Hello World!"}, {"2", "Goodbye World!"}, {"3", "Goodnight World!"} }; } } public class ListResourceBundleDemo { public static void main(String[] args) { // create a new MyResources instance MyResources mr = new MyResources(); // print the value for key 1 System.out.println("" + mr.handleGetObject("1")); } }
Output
Let us compile and run the above program, this will produce the following result.
Hello World!
Defining Content of ListResourceBundle of String, Object Pair Example
The following example shows the usage of Java ListResourceBundle getContents() method to add entries to the ListResourceBundle. We've created a ListResourceBundle object having contents an two dimensional array of String, Student pairs. Then few entries are added by overriding getContents() method and then a value is retrieved using key and is printed.
package com.tutorialspoint; import java.util.Enumeration; import java.util.ListResourceBundle; // create a class that extends to ListResourceBundle class MyResources extends ListResourceBundle { // get contents must be implemented protected Object[][] getContents() { return new Object[][] { {"1", new Student(1, "Julie")}, {"2", new Student(2, "Robert")}, {"3", new Student(3, "Adam")} }; } } public class ListResourceBundleDemo { public static void main(String[] args) { // create a new MyResources instance MyResources mr = new MyResources(); // print the value for key 1 System.out.println("" + mr.handleGetObject("1")); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
[ 1, Julie ]