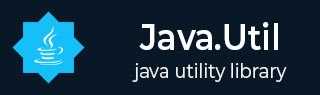
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java GregorianCalendar isLeapYear() Method
Description
The Java GregorianCalendar isLeapYear(int year) method determines if the given year is a leap year. Returns true if the given year is a leap year.
Declaration
Following is the declaration for java.util.GregorianCalendar.isLeapYear() method
public boolean isLeapYear(int year)
Parameters
year − the given year.
Return Value
This method returns true if the given year is a leap year; false otherwise.
Exception
NA
Checking an Year to be Leap Year Example
The following example shows the usage of Java GregorianCalendar isLeapYear(int) method. We're creating a GregorianCalendar instance of current date. We're checking if current year is leap year or not. Then we've checked another year for being a leap year.
package com.tutorialspoint; import java.util.GregorianCalendar; public class GregorianCalendarDemo { public static void main(String[] args) { // create a new calendar GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance(); // print the current date and time System.out.println("" + cal.getTime()); // check if it is a leap year boolean isLeapYear = cal.isLeapYear(cal.get(GregorianCalendar.YEAR)); System.out.println("Is leap year:" + isLeapYear); // check if 2012 is a leap year isLeapYear = cal.isLeapYear(2012); System.out.println("Is leap year:" + isLeapYear); } }
Output
Let us compile and run the above program, this will produce the following result −
Mon Apr 29 14:10:41 IST 2024 Is leap year:true Is leap year:true
Checking Future Year to be Leap Year Example
The following example shows the usage of Java GregorianCalendar isLeapYear(int) method. We're creating a GregorianCalendar instance of current date. We've added two years to the calendar instance. Then we've checked it for being a leap year.
package com.tutorialspoint; import java.util.GregorianCalendar; public class GregorianCalendarDemo { public static void main(String[] args) { // create a new calendar GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance(); // print the current date and time System.out.println("" + cal.getTime()); // add 2 years cal.add(GregorianCalendar.YEAR, 2); // check if it is a leap year boolean isLeapYear = cal.isLeapYear(cal.get(GregorianCalendar.YEAR)); System.out.println("Is leap year:" + isLeapYear); } }
Output
Let us compile and run the above program, this will produce the following result −
Mon Apr 29 14:11:02 IST 2024 Is leap year:false
Checking Past Year to be Leap Year Example
The following example shows the usage of Java GregorianCalendar isLeapYear(int) method. We're creating a GregorianCalendar instance of current date. We've subtracted two years from the calendar instance. Then we've checked it for being a leap year.
package com.tutorialspoint; import java.util.GregorianCalendar; public class GregorianCalendarDemo { public static void main(String[] args) { // create a new calendar GregorianCalendar cal = (GregorianCalendar) GregorianCalendar.getInstance(); // print the current date and time System.out.println("" + cal.getTime()); // subtract 2 years cal.add(GregorianCalendar.YEAR, -2); // check if it is a leap year boolean isLeapYear = cal.isLeapYear(cal.get(GregorianCalendar.YEAR)); System.out.println("Is leap year:" + isLeapYear); } }
Output
Let us compile and run the above program, this will produce the following result −
Mon Apr 29 14:11:22 IST 2024 Is leap year:false