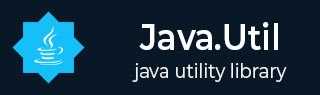
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections fill() Method
Description
The Java Collections fill(List<? super T>, T) method is used to replace all of the elements of the specified list with the specified element.
Declaration
Following is the declaration for java.util.Collections.fill() method.
public static <T> void fill(List<? super T> list, T obj)
Parameters
list − This is the list to be filled with the specified element.
obj − This is the element with which to fill the specified list.
Return Value
NA
Exception
UnsupportedOperationException − This is thrown if the specified list or its list-iterator does not support the set operation.
Replacing Values of List of Integers with Given Value Example
The following example shows the usage of Java Collection fill(List,T) method. We've created a List object with some integers, printed the original list. Using fill(List, T) method, we've replaced elements of the list with new value and then printed the updated list.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Integer> list = new ArrayList<>(Arrays.asList(1,2,3,4,5)); System.out.println("Initial collection value: " + list); // update values of this collection Collections.fill(list, 6); System.out.println("Final collection value: "+list); } }
Output
Let us compile and run the above program, this will produce the following result −
Initial collection value: [1, 2, 3, 4, 5] Final collection value: [6, 6, 6, 6, 6]
Replacing Values of List of Strings with Given Value Example
The following example shows the usage of Java Collection fill(List,T) method. We've created a List object with some strings, printed the original list. Using fill(List, T) method, we've replaced elements of the list with new value and then printed the updated list.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<String> list = new ArrayList<>(Arrays.asList("Welcome","to","Tutorialspoint")); System.out.println("Initial collection value: " + list); // update values of this collection Collections.fill(list, "TP"); System.out.println("Final collection value: "+list); } }
Output
Let us compile and run the above program, this will produce the following result −
Initial collection value: [Welcome, to, Tutorialspoint] Final collection value: [TP, TP, TP]
Replacing Values of List of Objects with Given Value Example
The following example shows the usage of Java Collection fill(List,T) method. We've created a List object with some Student objects, printed the original list. Using fill(List, T) method, we've replaced elements of the list with new value and then printed the updated list.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Student> list = new ArrayList<>(Arrays.asList(new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"))); System.out.println("Initial collection value: " + list); // update values of this collection Collections.fill(list, new Student(4, "Jene")); System.out.println("Final collection value: "+list); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
Initial collection value: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]] Final collection value: [[ 4, Jene ], [ 4, Jene ], [ 4, Jene ]]
To Continue Learning Please Login