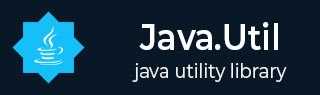
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Calendar setTimeInMillis() Method
Description
The Java Calendar setTimeInMillis(long) method sets Calendar's current time from the given long value.
Declaration
Following is the declaration for java.util.Calendar.setTimeInMillis() method
public void setTimeInMillis(long millis)
Parameters
millis − the new time in UTC milliseconds from the epoch.
Return Value
This method does not return a value.
Exception
NA
Setting Time in Milliseconds in a Current Dated Calendar Instance Example
The following example shows the usage of Java Calendar setTimeInMillis() method. We're creating an instance of a Calendar of current date using getInstance() method and printing the date and time using getTime() method. Then we're updating the date by using setTimeInMillis(). In the end, updated date is printed.
package com.tutorialspoint; import java.util.Calendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = Calendar.getInstance(); // get the time System.out.println("Current time is :" + cal.getTime()); // set time to 5000 ms after january 1 1970 cal.setTimeInMillis(5000); // print the new time System.out.println("After setting Time: " + cal.getTime()); } }
Output
Let us compile and run the above program, this will produce the following result −
Current time is :Wed Sep 28 17:56:54 IST 2022 After setting Time: Thu Jan 01 05:30:05 IST 1970
Setting Time in Milliseconds in a Current Dated GregorianCalendar Instance Example
The following example shows the usage of Java Calendar setTimeInMillis() method. We're creating an instance of a Calendar of current date using GregorianCalendar() method and printing the date and time using getTime() method. Then we're updating the date by setting it using setTimeInMillis(). In the end, updated date is printed.
package com.tutorialspoint; import java.util.Calendar; import java.util.GregorianCalendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = new GregorianCalendar(); // get the current time System.out.println("Current time is :" + cal.getTime()); // set time to 5000 ms after january 1 1970 cal.setTimeInMillis(5000); // print the new time System.out.println("After setting Time: " + cal.getTime()); } }
Output
Let us compile and run the above program, this will produce the following result −
Current time is :Wed Sep 28 17:59:23 IST 2022 After setting Time: Thu Jan 01 05:30:05 IST 1970
Setting Time in Milliseconds in a Given Dated GregorianCalendar Instance Example
The following example shows the usage of Java Calendar setTimeInMillis() method. We're creating an instance of a Calendar of particular date using GregorianCalendar() method and printing the date and time using getTime() method. Then we're updating the date by setting it using setTimeInMillis(). In the end, updated date is printed.
package com.tutorialspoint; import java.util.Calendar; import java.util.GregorianCalendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = new GregorianCalendar(2022,8,27); // get the current time System.out.println("Current time is :" + cal.getTime()); // set time to 5000 ms after january 1 1970 cal.setTimeInMillis(5000); // print the new time System.out.println("After setting Time: " + cal.getTime()); } }
Output
Let us compile and run the above program, this will produce the following result −
Current time is :Tue Sep 27 00:00:00 IST 2022 After setting Time: Thu Jan 01 05:30:05 IST 1970