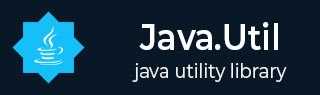
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Arrays stream(T[] a)Method
Description
The Java Arrays stream(T[] a) method returns a Stream covering all of the specified array.
Declaration
Following is the declaration for java.util.Arrays.stream(T[] a) method
public static <T> Stream<T> stream(T[] array)
Type Parameters
T − This is the type of elements.
Parameters
a − This is the array, assumed to be unmodified during use.
Return Value
This method returns a stream for the array elements.
Exception
NA
Java Arrays stream(T[] a, int fromIndex, int toIndex) Method
Description
The Java Arrays stream(T[] a, int fromIndex, int toIndex) method returns a Stream covering the specified range of the specified array.
Declaration
Following is the declaration for java.util.Arrays.stream(T[] a, int fromIndex, int toIndex) method
public static <T> Stream<T> stream(T[] array, int fromIndex, int toIndex)
Parameters
a − This is the array, assumed to be unmodified during use.
fromIndex − This is the index of the first element, inclusive, to be covered.
toIndex − This is the index of the last element, exclusive, to be covered
Return Value
This method returns a stream for the array elements.
Exception
ArrayIndexOutOfBoundsException − if fromIndex < 0 or toIndex > array.length
Getting stream of Array of Objects Example
The following example shows the usage of Java Arrays stream(T[]) method. First, we've created an array of student objects. Using stream() method, array is printed thereafter.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initialize array Student arr[] = { new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"), new Student(4, "Jene") }; System.out.print("Array: [ "); // use the stream to print each item Arrays.stream(arr).forEach(i -> System.out.print(i + " ")); System.out.print("]"); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
Array: [ [ 1, Julie ] [ 2, Robert ] [ 3, Adam ] [ 4, Jene ] ]
Getting stream of Array of Objects using Range Example
The following example shows the usage of Java Arrays stream(T[], int, int) method. First, we've created an array of student objects. Using stream() method, array is printed thereafter.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initialize array Student arr[] = { new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"), new Student(4, "Jene") }; System.out.print("Array: [ "); // use the stream to print each item Arrays.stream(arr, 0, arr.length).forEach(i -> System.out.print(i + " ")); System.out.print("]"); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
Array: [ [ 1, Julie ] [ 2, Robert ] [ 3, Adam ] [ 4, Jene ] ]
Getting stream of Sub-Array of Objects Example
The following example shows the usage of Java Arrays stream(T[], int, int) method. First, we've created an array of student objects. Using stream() method, a sub-array is printed thereafter.
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initialize array Student arr[] = { new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"), new Student(4, "Jene") }; System.out.print("Array: [ "); // use the stream to print each item Arrays.stream(arr, 0, 3).forEach(i -> System.out.print(i + " ")); System.out.print("]"); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
Array: [ [ 1, Julie ] [ 2, Robert ] [ 3, Adam ] ]
To Continue Learning Please Login