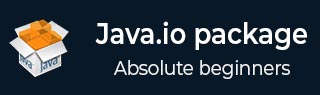
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java - ByteArrayInputStream class with Example
The java.io.ByteArrayInputStream class contains an internal buffer that contains bytes that may be read from the stream. An internal counter keeps track of the next byte to be supplied by the read method.Following are the important points about ByteArrayInputStream −
Closing a ByteArrayInputStream has no effect.
The methods in this class can be called after the stream has been closed without generating an IOException.
Class declaration
Following is the declaration for java.io.ByteArrayInputStream class −
public class ByteArrayInputStream extends InputStream
Field
Following are the fields for java.io.ByteArrayInputStream class −
protected byte[] buf − This is an array of bytes that was provided by the creator of the stream.
protected int count − This is the index one greater than the last valid character in the input stream buffer.
protected int mark − This is the currently marked position in the stream.
protected int pos − This is the index of the next character to read from the input stream buffer.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | ByteArrayInputStream(byte[] buf) This creates a ByteArrayInputStream so that it uses buf as its buffer array. |
2 | ByteArrayInputStream(byte[] buf, int offset, int length) This creates ByteArrayInputStream that uses buf as its buffer array. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | int available()
This method returns the number of remaining bytes that can be read (or skipped over) from this input stream. |
2 | void close()
Closing a ByteArrayInputStream has no effect. |
3 | void mark(int readAheadLimit)
This method set the current marked position in the stream. |
4 | boolean markSupported()
This method tests if this InputStream supports mark/reset. |
5 | int read()
This method reads the next byte of data from this input stream. |
6 | int read(byte[] b, int off, int len)
This method reads up to len bytes of data into an array of bytes from this input stream. |
7 | void reset()
This method resets the buffer to the marked position. |
8 | long skip(long n)
This method skips n bytes of input from this input stream. |
Methods inherited
This class inherits methods from the following classes −
- java.io.InputStream
- java.io.Object
Example
Following is the example to demonstrate ByteArrayInputStream and ByteArrayOutputStream.
import java.io.ByteArrayInputStream; import java.io.ByteArrayOutputStream; import java.io.IOException; public class ByteStreamTest { public static void main(String args[])throws IOException { ByteArrayOutputStream bOutput = new ByteArrayOutputStream(12); while( bOutput.size()!= 10 ) { // Gets the inputs from the user bOutput.write("hello".getBytes()); } byte b [] = bOutput.toByteArray(); System.out.println("Print the content"); for(int x = 0 ; x < b.length; x++) { // printing the characters System.out.print((char)b[x] + " "); } System.out.println(" "); int c; ByteArrayInputStream bInput = new ByteArrayInputStream(b); System.out.println("Converting characters to Upper case " ); for(int y = 0 ; y < 1; y++) { while(( c = bInput.read())!= -1) { System.out.println(Character.toUpperCase((char)c)); } bInput.reset(); } } }
Output
Print the content h e l l o h e l l o Converting characters to Upper case H E L L O H E L L O