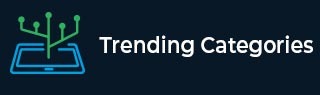
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java sql.Time toString() method with example
The toString() method of the java.sql.Time class returns the JDBC escape format of the time of the current Time object as String variable.
i.e. using this method you can convert a Time object to a String.
//Retrieving the Time object Time timeObj = rs.getTime("DeliveryTime"); //Converting the Time object to String format String time = timeObj.toString();
Example
Let us create a table with name dispatches in MySQL database using CREATE statement as follows −
CREATE TABLE dispatches( ProductName VARCHAR(255), CustomerName VARCHAR(255), DispatchDate date, DeliveryTime time, Price INT, Location VARCHAR(255));
Now, we will insert 5 records in dispatches table using INSERT statements −
insert into dispatches values('Key-Board', 'Raja', DATE('2019-09-01'), TIME('11:00:00'), 7000, 'Hyderabad'); insert into dispatches values('Earphones', 'Roja', DATE('2019-05-01'), TIME('11:00:00'), 2000, 'Vishakhapatnam'); insert into dispatches values('Mouse', 'Puja', DATE('2019-03-01'), TIME('10:59:59'), 3000, 'Vijayawada'); insert into dispatches values('Mobile', 'Vanaja', DATE('2019-03-01'), TIME('10:10:52'), 9000, 'Chennai'); insert into dispatches values('Headset', 'Jalaja', DATE('2019-04-06'), TIME('11:08:59'), 6000, 'Goa');
Following JDBC program establishes connection with the database and retrieves the contents of the dispatches table.
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.sql.Time; public class Time_toString { public static void main(String args[]) throws SQLException { //Registering the Driver DriverManager.registerDriver(new com.mysql.jdbc.Driver()); //Getting the connection String mysqlUrl = "jdbc:mysql://localhost/mydatabase"; Connection con = DriverManager.getConnection(mysqlUrl, "root", "password"); System.out.println("Connection established......"); //Creating a Statement object Statement stmt = con.createStatement(); //Query to retrieve the contents of the dispatches table String query = "select * from dispatches"; //Executing the query ResultSet rs = stmt.executeQuery(query); while(rs.next()) { System.out.println("Product Name: "+rs.getString("ProductName")); System.out.println("Customer Name: "+rs.getString("CustomerName")); System.out.println("Dispatch Date: "+rs.getDate("DispatchDate")); Time timeObj = rs.getTime("DeliveryTime"); //Converting the Time object to String format String time = timeObj.toString(); System.out.println("Delivery time in String format: "+time); System.out.println("Location: "+rs.getString("Location")); System.out.println(); } } }
Here, in this program while retrieving the column values we have converted the Delivery Time value from Time object to string format using the toString() method of the Time class and displayed it.
Output
Connection established...... Product Name: Key-Board Customer Name: Raja Dispatch Date: 2019-09-01 Delivery time in String format: 11:00:00 Location: Hyderabad Product Name: Earphones Customer Name: Roja Dispatch Date: 2019-05-01 Delivery time in String format: 11:00:00 Location: Vishakhapatnam Product Name: Mouse Customer Name: Puja Dispatch Date: 2019-03-01 Delivery time in String format: 10:59:59 Location: Vijayawada Product Name: Mobile Customer Name: Vanaja Dispatch Date: 2019-03-01 Delivery time in String format: 10:10:52 Location: Chennai Product Name: Headset Customer Name: Jalaja Dispatch Date: 2019-04-06 Delivery time in String format: 11:08:59 Location: Goa
Advertisements