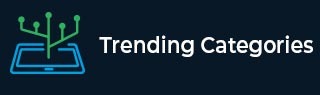
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to Toss a Coin
Tossing a coin is flipping a coin into the air and letting it fall back down. When you toss a coin, it's like a game where you can choose heads or tails, and the side that lands facing up is the result. This method is used when we want to make decisions or settle things randomly.
Problem Statement
Create a Java program to simulate the flipping of a coin 10 times, recording the number of times the result is "heads" and "tails".
Output
Chances = 10 Heads: 3 Tails: 7
Algorithms
Step-1: Create an instance of the "Toss" class. Initialize "heads" to 0, "tails" to 0 and set "chances" to 10.
Step-2: Define a method "chanceFunc" in the "Toss" class. Inside the "chanceFunc" create an instance of the Random class.
Step-3: Generate a random integer between 0 and 1 using r.nextInt(2). If the random integer is 1, return "tails" otherwise return "heads".
Step-4: In the main method of the "Demo" class a loop will run from i = 1 to i <= chances.
Step-5: Call the chanceFunc method, if the result is "tails" then increment the "tails" counter by 1. Else:Increment the "heads" counter by 1.
Step-6: Print the total number of "chances" , number of "heads", number of "tails".
Code Explanation
Let's say we have a coin and 10 chances. Here, we will first initialize the values for head, tail and chances -
int heads = 0; int tails = 0; int chances = 10;
Now, we will get the head and tail values using the Random object -
for (int i = 1; i<= chances; i++) { if (t.chanceFunc().equals("tails")) { tails++; } else { heads++; } }
Above, the function chanceFunc() is having Random class with the nextInt() method to get the next random value. The condition is checked and the heads and tails values are returned -
public String chanceFunc() { Random r = new Random(); int chance = r.nextInt(2); if (chance == 1) { return"tails"; } else { return"heads"; } }
Java Program to Toss a Coin
import java.util.Random; class Toss { public String chanceFunc() { Random r = new Random(); int chance = r.nextInt(2); if (chance == 1) { return "tails"; } else { return"heads"; } } } public class Demo { public static void main(String[] args) { Toss t = new Toss(); int heads = 0; int tails = 0; int chances = 10; for (int i = 1; i<= chances; i++) { if (t.chanceFunc().equals("tails")) { tails++; } else { heads++; } } System.out.println("Chances = " + chances); System.out.println("Heads: " + heads); System.out.println("Tails: " + tails); } }
Output
Chances = 10 Heads: 3 Tails: 7
Let us run the program again -
Chances = 10 Heads: 4 Tails: 6