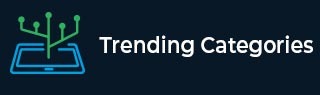
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to Segregate 0s on Left Side & 1s on Right Side of the Array
Segregation is a process in the field of software engineering where a code forced to depend on those methods which are not in use. The segregation interface is known as ISP. It splits the interfaces those are vast in nature. In a java environment there are lots of advantage to implement the segregation principal. It increases the readability of the particular code. As well as helps to maintain that particular code in a convenient manner.
Example: There is an array of 7s and 16s in a random manner. We have to segregate the 7s on the left side and 16s to the right. The basic goal is here to traverse those elements
Input array = [7,16,7,16,7,16,7,16] Output array = [7,7,7,7,16,16,16,16]
Let’s discuss on the implementation of the segregation process to perform 0s on left side & 1s on right side to an array.
Algorithm to Segregate 0s on Left Side & 1s on Right Side of the Array:-
Step 1 − START.
Step 2 − Build up the two different indexes.
Step 3 − Declare the first one as 0.(Left)
Step 4 − Declare the Second one as n-1.(Right)
Step 5 − Follow the condition: left<right.
Step 6 − Increment the whole index left( 0s at it)
Step 7 − Decrement the whole index right(1s at it)
Step 8 − If, left<right then exchange the position.
Step 9 − Else, carry forward the process.
Syntax
int left = 0, right = size-1; while (left < right){ while (array[left] == 0 && left < right) left++; while (array[right] == 1 && left < right) right--; if (left < right){ array[left] = 0; array[right] = 1; left++; right--; } }
In this syntax we can see how the function is segregating all the 0s on the left and 1s on right present in an array.
Take two pointers;
for element X starting from beginning (index = 0)
type1 (for element 1) starting from end (index = array.length-1).
Initialize type0 = 0
type1 = array.length-1
It is necessary to Put 1 to the right side of the array. After the process done, then 0 will definitely towards the left.
Approach
Approach 1 − By using the segregation method by counting
Approach 2 − By using the sorting method on an array
Approach 3 − By using the pointers
Approach 4 − By using Brute Force approach
By using the segregation method by counting
Process: Here is the step by step process of the segregation method by counting.
Count the 0s number.
Traverse the whole array.
Search for the elements.
Maintain the data and increment it until the result appears.
Print.
Remaining number of 1s.
Print remaining.
Here in this particular code the Time Complexity: O(n) and Auxiliary Space: O(1).
Example 1
import java.util.*; import java.util.Arrays; public class tutorialspoint { static void segregate7and16(int arr[], int n){ int count = 0; for (int a = 0; a < n; a++) { if (arr[a] == 0) count++; } for (int a = 0; a < count; a++) arr[a] = 0; for (int i = count; i < n; i++) arr[i] = 1; } static void print(int arr[], int n){ System.out.print("Array after segregation list is here "); for (int i = 0; i < n; i++) System.out.print(arr[i] + " "); } public static void main(String[] args){ int arr[] = new int[] { 7, 16, 7, 16, 16, 16,7,16 }; int n = arr.length; segregate7and16(arr, n); print(arr, n); } }
Output
Array after segregation list is here 1 1 1 1 1 1 1 1
By using a sorting method on an array
The sort() is a Java Until class method.
The Syntax is -
public static void sort(int[] arr, int from_Index, int to_Index)
Parameters:
arr- array to be sorted
from_Index - Inclusive to be sorted.
to_Index - exclisive to be sorted.
Exemple 2
import java.util.*; public class tutoriaspoint { static void print(int arr[], int n){ System.out.print("Array after segregation is "); for (int i = 0; i < n; ++i) System.out.print(arr[i] + " "); } public static void main(String[] args){ int arr[] = new int[] { 0, 1, 0, 1, 1, 1 }; int n = arr.length; Arrays.sort(arr); print(arr, n); } }
Output
Array after segregation is 0 0 1 1 1 1
By using pointers
It is needed to maintained left pointer and swap it with the position of the left. When zero is found in the array and increment the left pointer.
Example 3
import java.util.*; import java.io.*; public class tutorialspoint { static void print(int a[]){ System.out.print("Array after segregation is: "); for (int i = 0; i < a.length; ++i) { System.out.print(a[i] + " "); } } public static void main(String[] args){ int a[] = { 1, 1, 0, 0, 0, 0, 1, 1 }; int left = 0; for (int i = 0; i < a.length; ++i) { if (a[i] == 0) { int temp = a[left]; a[left] = a[i]; a[i] = temp; ++left; } } print(a); } }
Output
Array after segregation is: 0 0 0 0 1 1 1 1
By using Brute Force approach
Maintain left pointer and swap it with the position of the left. When zero found in the array and increment the left pointer.
The method will count the number first. Then it will fill the data accordingly with 0s and 1s.
Process:
The process will count the number according the array size.
After counting the filling will start.
Example 4
public class segregatearray { static void segregating0sand1s(int arr[], int n){ int count = 0; //count no of zeros in array for (int i = 0; i < n; i++) { if (arr[i] == 0) count++; } for (int i = 0; i < count; i++) arr[i] = 0; // fill the array with 0 until termination for (int i = count; i < n; i++) arr[i] = 1; // fill remaining array with 1 until termination } static void print(int arr[], int n){ System.out.print("Array after the process 0s and 1s are "); for (int i = 0; i < n; i++) System.out.print(arr[i] + " "); } public static void main(String[] args){ int arr[] = new int[]{ 1, 1, 0, 0, 1, 1 }; int n = arr.length; segregating0sand1s(arr, n); print(arr, n); } }
Output
Array after the process 0s and 1s are 0 0 1 1 1 1
Conclusion
In this article, we have learnt how to segregate all 0s on left and all 1s on right as segregate 0 and 1 along with its different approaches, pseudocode, implementation, and code. Here we have defined an array and pass that array along with its total length to the function.