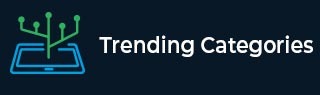
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to return a Date set to noon to the closest possible millisecond of the day
Use the getMinimum() method in Java to returns the minimum value for the given calendar field. We will use it to set the minute, second and millisecond.
Let us first declare a calendar object.
Calendar dateNoon = Calendar.getInstance();
Now, we will set the hour, minute, second and millisecond to get the closest possible millisecond of the day.
dateNoon.set(Calendar.HOUR_OF_DAY, 12); dateNoon.set(Calendar.MINUTE, dateNoon.getMinimum(Calendar.MINUTE)); dateNoon.set(Calendar.SECOND, dateNoon.getMinimum(Calendar.SECOND)); // millisecond dateNoon.set(Calendar.MILLISECOND, dateNoon.getMinimum(Calendar.MILLISECOND));
Example
import java.util.Calendar; import java.util.GregorianCalendar; public class Demo { public static void main(String[] argv) throws Exception { Calendar dateNoon = Calendar.getInstance(); // hour, minute and second dateNoon.set(Calendar.HOUR_OF_DAY, 12); dateNoon.set(Calendar.MINUTE, dateNoon.getMinimum(Calendar.MINUTE)); dateNoon.set(Calendar.SECOND, dateNoon.getMinimum(Calendar.SECOND)); // millisecond dateNoon.set(Calendar.MILLISECOND, dateNoon.getMinimum(Calendar.MILLISECOND)); System.out.println(dateNoon.getTime()); } }
Output
Fri Nov 23 12:00:00 UTC 2018
Advertisements