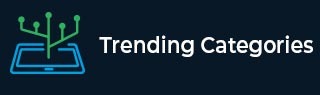
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to print the diamond shape
A diamond shape can be printed by printing a triangle and then an inverted triangle. An example of this is given as follows −
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
A program that demonstrates this is given as follows.
Example
public class Example { public static void main(String[] args) { int n = 6; int s = n - 1; System.out.print("A diamond with " + 2*n + " rows is as follows:
"); for (int i = 0; i < n; i++) { for (int j = 0; j < s; j++) System.out.print(" "); for (int j = 0; j <= i; j++) System.out.print("* "); System.out.print("
"); s--; } s = 0; for (int i = n; i > 0; i--) { for (int j = 0; j < s; j++) System.out.print(" "); for (int j = 0; j < i; j++) System.out.print("* "); System.out.print("
"); s++; } } }
Output
A diamond with 12 rows is as follows: * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
Now let us understand the above program.
The diamond shape is created by printing a triangle and then an inverted triangle. This is done by using nested for loops. The code snippet for the upper triangle is given as follows.
int n = 6; int s = n - 1; System.out.print("A diamond with " + 2*n + " rows is as follows:
"); for (int i = 0; i < n; i++) { for (int j = 0; j < s; j++) System.out.print(" "); for (int j = 0; j <= i; j++) System.out.print("* "); System.out.print("
"); s--; }
The code snippet for the inverted lower triangle is given as follows.
s = 0; for (int i = n; i > 0; i--) { for (int j = 0; j < s; j++) System.out.print(" "); for (int j = 0; j < i; j++) System.out.print("* "); System.out.print("
"); s++; }
Advertisements