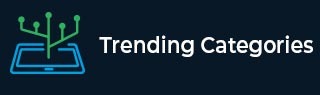
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to Illustrate Escaping Characters in Regex
Here, we will demonstrate escaping characters in Regex through Java Program. Before diving deep into the topic, let us get acquainted with the term Escaping Characters and Regex.
Regex
It is an acronym for a Regular expression. It is an API that offers users to define String patterns that are useful for finding, modifying, and editing strings. A couple of areas of strings where Regex is frequently used to define the limitations include email validation and passwords. The java.util.regex package encompasses regular expressions.
Escape character
When a character is preceded by a backslash (\), it includes numbers, alphabets, and punctuation marks. The compiler treats these characters differently and such a character is termed an escape character.
Some of its examples include:
\n - It is used to add a new line in the text in this instance.
\’ - It is used to add a single quote character in the text at this instance.
Methods to escape characters
To match in the regular expression, special characters like dot (.), hash (#), etc., that have a special significance to the regular expression must be escaped.
For instance, if dot(.) in a regular expression is not escaped, it matches any single character and produces unclear results.
In Java Regex, characters can be escaped in two different ways, which we shall study in detail below.
Using \Q and \E to escape
Using the backslash (\) to escape
Method 1. Using Q and E to escape
To escape characters, we can employ the Q and E escape sequences.
The escape sequence begins with the letter Q and ends with the letter E.
Between the letters Q and E, all characters escape.
commonly employed to escape numerous characters.
Example 1
The following program demonstrates the working of an escape character in regex using a dot.
// Java Program to demonstrate how to escape characters in Java // Regex Using \Q and \E for escaping import java.io.*; import java.util.regex.*; //creation of a class named Regexeg1 public class Regexeg1 { // Main method public static void main(String[] args) { // providing two strings as inputs String s1 = "Tutorials.point"; String s2 = "Tutorialspoint"; //creation of an object of Pattern class with dot escaped Pattern p1 = Pattern.compile("\\Q.\\E"); //creation of an object of Pattern class without escaping the dot Pattern p2 = Pattern.compile("."); // Matchers for every combination of patterns and strings Matcher m1 = p1.matcher(s1); Matcher m2 = p1.matcher(s2); Matcher m3 = p2.matcher(s1); Matcher m4 = p2.matcher(s2); // find whether p1 and p2 match and display the Boolean value as a result System.out.println("p1 matches s1: " + m1.find()); System.out.println("p1 matches s2: " + m2.find()); System.out.println("p2 matches s1: " + m3.find()); System.out.println("p2 matches s2: " + m4.find()); } }
Output
p1 matches s1: true p1 matches s2: false p2 matches s1: true p2 matches s2: true
In the above Java program, the usage of \Q and \E in regular expressions to escape a string of characters is demonstrated.
Two input strings namely s1 and s2, and two Pattern objects namely, p1 and p2 are created where p1 uses \Q and \E to escape the dot character ".", and p2 does not escape the dot character.
Four Matcher objects namely m1, m2, m3, and m4, which are used to match the input strings with the Pattern objects are created.
Finally, the program displays the Boolean value true if the Pattern objects p1 and p2 match the input strings s1 and s2 using the Matcher objects m1, m2, m3, and m4 and displays the Boolean value false if it does not match.
Method 2. Using the backslash (//) as an escape character
A backslash can be used to escape characters.
Since the backslash character is a character of its own, we need two backslashes.
Then, the characters are escaped.
Characters at the end of the string are often escaped using it.
Example 2
The following program demonstrates the working of an escape character in regex using a backslash (//).
// Java Program to demonstrate how to escape characters in Java
// Regex using backslash (\) for escaping
import java.io.*;
import java.util.regex.*;
//creation of a class named Regexeg2
public class Regexeg2 {
public static void main (String[] args) {
// providing two strings as inputs
String s1="Tutorials.point";
String s2="Tutorialspoint";
//creation of an object of Pattern class with dot escaped
Pattern p1=Pattern.compile("\\.");
//creation of an object of Pattern class without dot escaped
Pattern p2=Pattern.compile(".");
//Four matchers for each pattern string combination
Matcher m1=p1.matcher(s1);
Matcher m2=p1.matcher(s2);
Matcher m3=p2.matcher(s1);
Matcher m4=p2.matcher(s2);
// find whether p1 and p2 match and display the boolean value as a result
System.out.println("p1 matches s1: "+m1.find());
System.out.println("p1 matches s2: "+m2.find());
System.out.println("p2 matches s1: "+m3.find());
System.out.println("p2 matches s2: "+m4.find());
}
}
Output
p1 matches s1: true p1 matches s2: false p2 matches s1: true p2 matches s2: true
In the above Java code, the usage of escaping characters in regular expressions is demonstrated using backslash to match a special character in a string.
Here, two input strings namely s1 and s2, and two Pattern objects p1 and p2 are being created where p1 has the dot character "." escaped by a backslash, and p2 does not escape the dot character.
Four Matcher objects namely m1, m2, m3, and m4 are then created to match the input strings with the Pattern objects.
Finally, the program displays the Boolean value true if the Pattern objects p1 and p2 match the input strings s1 and s2 using the Matcher objects m1, m2, m3, and m4 and displays a Boolean value false if they do not match.
This article threw light on the methods to escape characters in Regex. The article began with a discussion of the term regex and Escape characters Two methods along with their implementations are discussed here to have a clear understanding of the topic.