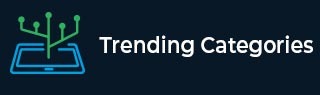
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to display table with columns in the output using Formatter
To display table with columns as output, use the Formatter class. For working with Formatter class, import the following package −
import java.util.Formatter;
Consider an array with some elements. This is the array which we will be shown as output in tabular form −
double arr[] = { 1.7, 2.5, 3.1, 4.5, 5.7, 6.9, 7.7, 8.9, 9.1 };
While displaying the double array values, use the %f to set spaces −
for (double d : arr) { f.format("%14.2f %14.2f %15.2f
", d, Math.ceil(d), Math.floor(d)); }
The following is an example −
Example
import java.util.Formatter; public class Demo { public static void main(String[] argv) throws Exception { double arr[] = { 1.7, 2.5, 3.1, 4.5, 5.7, 6.9, 7.7, 8.9, 9.1 }; Formatter f = new Formatter(); f.format("%15s %15s %15s
", "Points1", "Points2", "Points3"); System.out.println("The point list...
"); for (double d : arr) { f.format("%14.2f %14.2f %15.2f
", d, Math.ceil(d), Math.floor(d)); } System.out.println(f); } }
Output
The point list... Points1 Points2 Points3 1.70 2.00 1.00 2.50 3.00 2.00 3.10 4.00 3.00 4.50 5.00 4.00 5.70 6.00 5.00 6.90 7.00 6.00 7.70 8.00 7.00 8.90 9.00 8.00 9.10 10.00 9.00
Advertisements