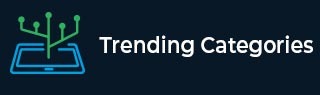
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java program to count the characters in each word in a given sentence
To count the characters in each word in a given sentence, the Java code is as follows −
Example
import java.util.*; public class Demo{ static final int max_chars = 256; static void char_occurence(String my_str){ int count[] = new int[max_chars]; int str_len = my_str.length(); for (int i = 0; i < str_len; i++) count[my_str.charAt(i)]++; char ch[] = new char[my_str.length()]; for (int i = 0; i < str_len; i++){ ch[i] = my_str.charAt(i); int find = 0; for (int j = 0; j <= i; j++){ if (my_str.charAt(i) == ch[j]) find++; } if (find == 1) System.out.println("The number of occurrence of " + my_str.charAt(i) + " is :" + count[my_str.charAt(i)]); } } public static void main(String[] args){ Scanner my_scan = new Scanner(System.in); String my_str = "This is a sample"; char_occurence(my_str); } }
Output
The number of occurrence of T is :1 The number of occurrence of h is :1 The number of occurrence of i is :2 The number of occurrence of s is :3 The number of occurrence of is :3 The number of occurrence of a is :2 The number of occurrence of m is :1 The number of occurrence of p is :1 The number of occurrence of l is :1 The number of occurrence of e is :1
A class named Demo contains the function ‘char_occurence’ that iterates through the string and counts every character and assigns its count to the respective character in the count array. In the main function, a Scanner class object is created to read the input from the console. The function is called on string and the count of every character is displayed on the console.
Advertisements