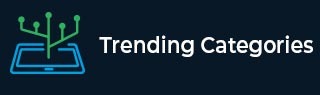
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to Count set bits in an integer
To count set bits in an integer, the Java code is as follows −
Example
import java.io.*; public class Demo{ static int set_bits_count(int num){ int count = 0; while (num > 0){ num &= (num - 1); count++; } return count; } public static void main(String args[]){ int num =11; System.out.println("The number of set bits in 11 is "); System.out.println(set_bits_count(num)); } }
Output
The number of set bits in 11 is 3
The above is an implementation of the Brian Kernighan algorithm. A class named Demo contains a static function named ‘set_bits_count’. This function checks if the number is 0, and if not, assigns a variable named ‘count’ to 0. It performs the ‘and’ operation on the number and the number decremented by 1.
Next, the ‘count’ value is decremented after this operation. In the end, the count value is returned. In the main function, the value whose set bits need to be found is defined. The function is called by passing the number as a parameter. Relevant messages are displayed on the console.
Advertisements