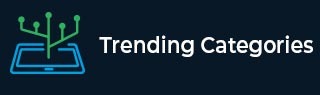
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to count letters in a String
Let’s say we have the following string, that has some letters and numbers.
String str = "9as78";
Now loop through the length of this string and use the Character.isLetter() method. Within that, use the charAt() method to check for each character/ number in the string.
for (int i = 0; i < str.length(); i++) { if (Character.isLetter(str.charAt(i))) count++; }
We have set a count variable above to get the length of the letters in the string.
Here’s the complete example.
Example
public class Demo { public static void main(String []args) { String str = "9as78"; int count = 0; System.out.println("String: "+str); for (int i = 0; i < str.length(); i++) { if (Character.isLetter(str.charAt(i))) count++; } System.out.println("Letters: "+count); } }
Output
String: 9as78 Letters: 2
Let us see another example.
Example
public class Demo { public static void main(String []args) { String str = "amit"; int count = 0; System.out.println("String: "+str); for (int i = 0; i < str.length(); i++) { if (Character.isLetter(str.charAt(i))) count++; } System.out.println("Letters: "+count); } }
Output
String: amit Letters: 4
Advertisements