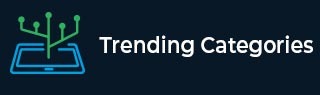
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to convert first character uppercase in a sentence
In order to convert first character as upper case in a sentence we have to first separate each word of sentence and then make first character of each word in upper case.After which we have to again join each word separated by space to make again sentence.
Now let take each task one by one.First in order to get each word of the sentence separately we would use Scanner class of Java and implement its hasnext method to check whether our sentence has any more word or not,in case word is present then we would get that word by using next method of same class.
After getting each word of the sentence we would now convert first character of each word in upper case by using toUpperCase method of Character class and join other characters of the word by using sub string method of string class and also append space at last of each word in order to make them again in a sentence.
Example
import java.util.Scanner; public class UpperCaseOfSentence { public static void main(String[] args) { String upper_case_line = ""; String str = "ram is a good boy."; Scanner lineScan = new Scanner(str); while(lineScan.hasNext()) { String word = lineScan.next(); upper_case_line += Character.toUpperCase(word.charAt(0)) + word.substring(1) + " "; } System.out.println(upper_case_line); } }
Output
Ram Is A Good Boy.