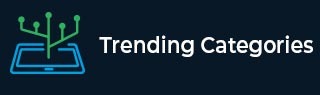
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to check whether the entered character a digit, white space, lower case or upper case character
To check whether the entered character is a digit, whitespace, lowercase or uppercase, you need to check for the ASCII values.
Let’s say we have a value in variable “val”, which is to be checked.
For Lower Case.
if(val >= 97 && val <= 123) { System.out.println("Lower Case"); }
For Upper Case
else if(val >= 65 && val <= 96) { System.out.println("Upper Case"); }
For Digit
else if(val >= 48 && val <= 57) { System.out.println("Digit"); }
Check for Whitespace now
else if(Character.isWhitespace(val)) { System.out.println("Whitespace"); }
The following is the complete example.
Example
public class Demo { public static void main(String []args) { char val ='L'; System.out.println("Given Value: "+val); if(val >= 97 && val <= 123) { System.out.println("Lower Case"); } else if(val >= 65 && val <= 96) { System.out.println("Upper Case"); } else if(val >= 48 && val <= 57) { System.out.println("Digit"); } else if(Character.isWhitespace(val)) { System.out.println("Whitespace"); } } }
Output
Given Value: L Upper Case
Let us see another example.
Example
public class Demo { public static void main(String []args) { char val ='L'; System.out.println("Given Value: "+val); if(val >= 97 && val <= 123) { System.out.println("Lower Case"); } else if(val >= 65 && val <= 96) { System.out.println("Upper Case"); } else if(val >= 48 && val <= 57) { System.out.println("Digit"); } else if(Character.isWhitespace(val)) { System.out.println("Whitespace"); } } }
Output
Given Value: a Lower Case
Advertisements