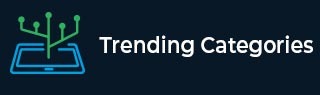
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to check whether the character is ASCII 7 bit printable
To check whether the entered value is ASCII 7-bit printable, check whether the characters ASCII value is greater than equal to 32 and less than 127 or not. These are the control characters.
Here, we have a character.
char one = '^';
Now, we have checked a condition with if-else for printable characters.
if (c >= 32 && c < 127) { System.out.println("Given value is printable!"); } else { System.out.println("Given value is not printable!"); }
Example
public class Demo { public static void main(String []args) { char c = '^'; System.out.println("Given value = "+c); if (c >= 32 && c < 127) { System.out.println("Given value is printable!"); } else { System.out.println("Given value is not printable!"); } } }
Output
Given value = ^ Given value is printable!
Let us see another example wherein the given value is a character.
Example
public class Demo { public static void main(String []args) { char c = 'y'; System.out.println("Given value = "+c); if ( c >= 32 && c < 127) { System.out.println("Given value is printable!"); } else { System.out.println("Given value is not printable!"); } } }
Output
Given value = y Given value is printable!
Advertisements