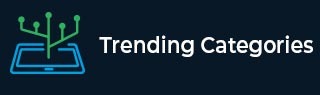
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java program to check if binary representation is palindrome
A palindrome is a sequence that is same both forwards and backwards. The binary representation of a number is checked for being a palindrome but no leading 0’s are considered. An example of this is given as follows −
Number = 5 Binary representation = 101
The binary representation of 5 is a palindrome as it is the same both forwards and backwards.
A program that demonstrates this is given as follows.
Example
public class Example { public static void main(String argc[]) { long num = 5, n1; long reverse = 0; n1 = num; while (n1 > 0) { reverse <<= 1; if ((n1 & 1) == 1) reverse ^= 1; n1 >>= 1; } if(num == reverse) { System.out.println("Binary representation of " + num + " is palindrome"); }else { System.out.println("Binary representation of " + num + " is not palindrome"); } } }
Output
Binary representation of 5 is palindrome
Now let us understand the above program.
The reverse of the given number 5 is obtained using a while loop. The code snippet that demonstrates this is given as follows −
long num = 5, n1; long reverse = 0; n1 = num; while (n1 > 0) { reverse <<= 1; if ((n1 & 1) == 1) reverse ^= 1; n1 >>= 1; }
If the number is same as its reverse, it is palindrome and that is printed, otherwise it is not palindrome and that is printed. The code snippet that demonstrates this is given as follows −
if(num == reverse) { System.out.println("Binary representation of " + num + " is palindrome"); }else { System.out.println("Binary representation of " + num + " is not palindrome"); }
Advertisements