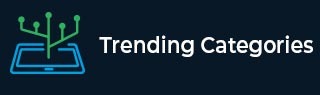
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program to check if any String in the list starts with a letter
First, create a List with String elements:
List<String> myList = new ArrayList<>(); myList.add("pqr"); myList.add("stu"); myList.add("vwx"); myList.add("yza"); myList.add("bcd"); myList.add("efg"); myList.add("vwxy");
Use the startsWith() method to check if any of the above string in the myList begins with a specific letter:
myList.stream().anyMatch((a) -> a.startsWith("v"));
TRUE is returned if any of the string begins with the specific letter, else FALSE.
The following is an example to check if any String in the list starts with a letter:
Example
import java.util.ArrayList; import java.util.List; public class Demo { public static void main(final String[] args) { List<String> myList = new ArrayList<>(); myList.add("pqr"); myList.add("stu"); myList.add("vwx"); myList.add("yza"); myList.add("bcd"); myList.add("efg"); myList.add("vwxy"); boolean res = myList.stream().anyMatch((a) -> a.startsWith("v")); System.out.println("Do any string begins with letter v = "+res); } }
Output
Do any string begins with letter v = true
Advertisements