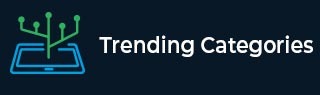
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program for Reversal algorithm for right rotation of an array
Array is a linear data structure that is used to store group of elements with similar datatypes. It stores data in a sequential manner. Once we create an array we can’t change its size i.e. it can store fixed number of elements.
This article will help you to understand the Reversal Algorithm and also, we will make a java program in which we create an array and perform right rotation by applying reversal algorithm.
Right Rotation of an Array
Let’s understand the term right rotation in context of an array.
In right rotation of an array, we simply shift the elements of the array to our right till the specified number of rotations.
Example 1

Example 2

In the above examples, when we are rotating the array 2 times the element from 0th position get shifted to 2nd position onwards and the last 2 elements get filled by first two positions.
When we are rotating the array 4 times the element from 0th position get shifted to 4th position onwards.
Syntax to declare an array
Data_Type nameOfarray[]; // declaration Or, // declaration with size Data_Type nameOfarray[] = new Data_Type[sizeofarray];
We can use any of the above syntax in our program.
Reversal Algorithm
The approach for reversal algorithm is as follows −
Step1 − First, we will reverse the given array from first index to last index.
Step2 − moving ahead we reverse the given array from first index to rt – 1 where rt is the number of times the rotation is required.
Step3 − In the last step, we will reverse the remaining array i.e. from rt to last index.
Note that for shifting the elements of array we will perform the swapping between them.
Program for right rotation using Reversal Algorithm
We will put our logic in user-defined method. Let’s discuss how we can create a user-defined method.
Syntax
accessSpecifier nonAccessModifier return_Type nameOfmethod(Parameters) { // your code will come here }
accessSpecifier − It is used to set the accessibility of the method. It may be public, protected, default and private.
nonAccessModifier − It shows additional functionalities or behaviour of the method such as static and final.
return_Type − The datatype a method is going to return. We use void keyword when method does not return anything.
nameOfmethod − Name of the method.
parameters − It contains name of the variable followed by datatype.
Example
public class Rotation { public void rev(int rot_arr[], int first, int last) { while(first < last) { int temp = rot_arr[first]; rot_arr[first] = rot_arr[last]; rot_arr[last] = temp; first++; last--; } } public int[] rotates(int rot_arr[], int rt) { rt = rt % rot_arr.length; rev(rot_arr, 0, rot_arr.length - 1); rev(rot_arr, 0, rt - 1); rev(rot_arr, rt, rot_arr.length - 1); return rot_arr; } public static void main(String[] args) { Rotation obj = new Rotation(); int rot_arr[] = {5, 8, 2, 4, 7, 1}; int rt = 4; System.out.print(" The given array is: "); for(int i = 0; i < rot_arr.length; i++) { System.out.print(rot_arr[i] + " "); } obj.rotates(rot_arr, rt); System.out.println(); System.out.print(" The given array after right rotation is: "); for(int i = 0; i < rot_arr.length; i++) { System.out.print(rot_arr[i] + " "); } } }
Output
The given array is: 5 8 2 4 7 1 The given array after right rotation is: 2 4 7 1 5 8
In the above code, we have created a class ‘Rotation’ and in that class we have taken two parameterized method ‘rev’ along with 3 parameters and ‘rotates’ with 2 parameters. The method ‘rev’ has been used for swapping elements and ‘rotates’ to apply the logic of reversal algorithm. In the main() method, we have created an object ‘obj’ of class ‘Rotation’ and using this object we have called ‘rotates’ method with two arguments.
Conclusion
In this article, we have understood what is right rotation and discussed the reversal algorithm. We have made a java program for right rotation of an array using reversal algorithm.