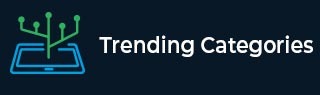
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java program for Hexadecimal to Decimal Conversion
The number system is of four types: Binary, Octal, Decimal and Hexadecimal with base value 2, 8, 10 and 16 respectively. The base value depends on the number of digits contained by number system. For example, binary number system contains only two digits 0 and 1. Hence, its base is 2.
In this article, we will discuss the hexadecimal and decimal number systems. Also, we will make java programs to convert hexadecimal to decimal numbers.
Hexadecimal and Decimal number system
Hexadecimal number system
It represents the numbers from 0 to 9 and A to F. There is a total of 16 numbers available in it and its base value is also 16. A single digit has a weight of power 16 and every digit is 16 times more weighty than previous one. 12A16, 34B16, 45C16 are a few examples of hexadecimal. In computers, the code for colors is generally written in hexadecimal form.
Suppose, we have to store a large decimal value if we store it in binary numbering system then binary string may become very long. In this situation, we can use hexadecimal number system that can store 4 bits of binary into 1 bit. It shortens the length of bits.
Decimal number system
It is the most used number system. It has 10 digits from 0 to 9. Hence, its base value is 10. If base value of a number is not mentioned then, it is considered to be 10. A single digit has a weight of power 10 and every digit is 10 times more weighty than previous one. For example, 1010, 43110, 98010 etc.
The following table represents the binary and decimal value of all hexdecimal digits −
Binary |
Decimal |
Hexadecimal |
---|---|---|
0001 |
1 |
1 |
0010 |
2 |
2 |
0011 |
3 |
3 |
0100 |
4 |
4 |
0101 |
5 |
5 |
0110 |
6 |
6 |
0111 |
7 |
7 |
1000 |
8 |
8 |
1001 |
9 |
9 |
1010 |
10 |
A |
1011 |
11 |
B |
1100 |
12 |
C |
1101 |
13 |
D |
1110 |
14 |
E |
1111 |
15 |
F |
Hexadecimal to Decimal Conversion
Let’s understand how we can convert hexadecimal to decimal.
Example 1
Convert hexadecimal (54A)16 to Decimal −
We can convert it to decimal by multiplying each digit with 16n-1 where n is the number of digits.
(54A)16 = 5 * 163-1 + 4 * 162-1 + A * 161-1
= 5 * 162 + 4 * 161 + 10 * 160 [A = 10 in decimal look at the table]
= 5 * 256 + 64 + 10 [160 is 1]
= 1280 + 74
= 1354
Now, we will see the java program in which we will apply the above logic to convert hexadecimal to decimal.
Approach 1: Using parseInt() method
It is a static method of ‘Integer’ class that returns a decimal value according to the specified base. It is available in ‘java.lang’ package.
Syntax
Integer.parseInt("String", base);
Parameters
String − the value that is going to convert
Base − given value is converted according to the given base
Example
public class Conversion { public static void main(String args[]) { // Converting and storing hexadecimal value to dec1 and dec2 with base 16 int dec1 = Integer.parseInt("54A", 16); int dec2 = Integer.parseInt("41C", 16); System.out.println("Decimal value of given Hexadecimal: " + dec1); System.out.println("Decimal value of given Hexadecimal: " + dec2); } }
Output
Decimal value of given Hexadecimal: 1354 Decimal value of given Hexadecimal: 1052
Approach 2: Using user-defined method
In this approach, we will make a user-defined method cnvrt() along with a parameter ‘hexNum’. We will declare and initialize ‘hexStr’ which will store all the hexadecimal digits in the form of a string. Then, we will run a for loop till the length of parameter ‘hexNum’. In that loop, we will fetch the characters and their index from ‘hexStr’ and then we will apply the logic of conversion.
In the main method, we will call the method ‘cnvrt()’ with different arguments.
Example
public class Conversion { public static void cnvrt(String hexNum) { // storing all the hexadecimal digits to this string String hexStr = "0123456789ABCDEF"; // converting given argument to uppercase hexNum = hexNum.toUpperCase(); int dec = 0; for (int i = 0; i < hexNum.length(); i++) { char ch = hexNum.charAt(i); // fetching characters sequentially int index = hexStr.indexOf(ch); // fetching index of characters dec = 16 * dec + index; // applying the logic of conversion } System.out.println("Decimal value of given Hexadecimal: " + dec); } public static void main(String args[]) { // calling the function with arguments cnvrt("54A"); cnvrt("41C"); } }
Output
Decimal value of given Hexadecimal: 1354 Decimal value of given Hexadecimal: 1052
Conclusion
In this article, we have understood the types of number system. These number systems serve as the base of any mathematical operation. Also, discussed two approaches for making java program to convert hexadecimal numbers to decimal numbers.