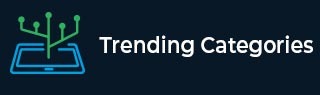
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Program for Binary Insertion Sort
Binary insertion sort uses the binary search to find the right position to insert an element at a specific index at every iteration. First, the location where the element needs to be inserted is found. Then, the elements are shifted to the next right location. Now, the specific element is placed in the position.
Problem Statement
Given an integer of array implement binary insertion sort using Java to sort it. The algorithm should use binary search to determine the correct position for each element and to maintain the sorted order it will shift elements accordingly.
Steps for Binary Insertion Sort
Below are the steps for binary insertion sort -
- Step 1: Initialize with implementing the binary search.
- Step 2: To make the space for current element we will shift the elements to right.
- Step 3: Inserting the current element at the determined position.
- Step 4: Print both before and after sorted array .
Binary Search Algorithm
Binary Search algorithm is an interval searching method that performs the searching in intervals only.
- Step 1: To search the key value we will select the middle value in the array and compare it with the key, if it matched return the position of it.
- Step 2: If the key value does not match with the middle value check whether the key value is greater or smaller than the mid value.
- Step 3: If the key value is greater, perform the search in the right sub-array; but if the key is lower than the mid value, perform the search in the left sub-array.
- Step 4: Repeat Steps 1, 2 and 3 iteratively, until the size of sub-array becomes 1.
- Step 5: If the key value is not found within the array, return a value that will indicate that the search was unsuccessful.
Java Program for Binary Insertion Sort
Following is the Java code for Binary Insertion Sort −
public class Demo { void Cocktail_Sort(int my_arr[]) { boolean swapped = true; int start = 0; int end = my_arr.length; while (swapped == true) { swapped = false; for (int i = start; i < end - 1; ++i) { if (my_arr[i] > my_arr[i + 1]) { int temp = my_arr[i]; my_arr[i] = my_arr[i + 1]; my_arr[i + 1] = temp; swapped = true; } } if (swapped == false) break; swapped = false; end = end - 1; for (int i = end - 1; i >= start; i--) { if (my_arr[i] > my_arr[i + 1]) { int temp = my_arr[i]; my_arr[i] = my_arr[i + 1]; my_arr[i + 1] = temp; swapped = true; } } start = start + 1; } } void print_values(int my_arr[]) { for (int i = 0; i < my_arr.length; i++) System.out.print(my_arr[i] + " "); System.out.println(); } public static void main(String[] args) { Demo my_object = new Demo(); int my_arr[] = { 6, 8, 34, 21, 0, 1, 98, 64, 6}; System.out.println("The array contains "); for (int i = 0; i < my_arr.length; i++) System.out.print(my_arr[i] + " "); System.out.println(); my_object.Cocktail_Sort(my_arr); System.out.println("The array after implementing cocktail sort is : "); my_object.print_values(my_arr); } }
Output
The array contains 6 8 34 21 0 1 98 64 6 The array after implementing cocktail sort is : 0 1 6 6 8 21 34 64 98
Code Explanation
The class Demo is performing the cocktail sort and prints the value of array. The main method initializes the Demo class and an integer array my_arr with values. It prints the original array, calls the Cocktail_Sort method to sort the array, and then prints the sorted array.
The method Cocktail_Sort begins by initializing a boolean variable swapped to true, and sets start to 0 and end to the length of the array.
The while loop continues as long as swapped is true. Initially, swapped is set to false at the beginning of each iteration.
A for loop iterates from the start index to end - 1. During each iteration, adjacent elements are compared. If an element is greater than the next one, they are swapped, and swapped is set to true.
If no elements were swapped in the forward pass, the array is already sorted, and the loop breaks.
If elements were swapped, swapped is set to false again, and end is decremented by 1. Another for loop runs from end - 1 to start. If an element is greater than the next one, they are swapped, and swapped is set to true.
After the backward pass, start is incremented by 1. The loop then repeats until no elements are swapped in both forward and backward passes.
The print_values method takes an array and prints its elements in a single line.