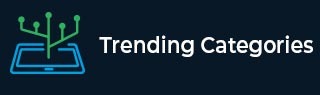
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java Nested Loops with Examples
Loops in java are called as control statements because they decide the flow of execution of a program based on some condition. Java allows the nesting of loops, when we put a loop within another loop then we call it a nested loop. Nested loops are very useful when we need to iterate through a matrix array and when we need to do any pattern-based questions.
In this article, we are going to learn about java nested loops with examples.
Nested Loops
We can create nested loops for the following control statements −
For loop
If else
While
Do while
For each
Let’s discuss these nested loops with some examples
Nested For loop
It is the most used control statement in any programming language because it is easy to understand and implement. Loop iterate till the given condition is true.
Syntax
for (initial expression; conditional expression; increment/decrement expression){ // code to be executed for (initial expression; conditional expression; increment/decrement expression) { // code to be executed } }
initial expression − executed once when loop begins.
conditional expression − code will be executed till conditional expression is true.
increment/decrement expression − to increment/decrement loop variable.
Example
The following program will perform addition of two matrices using nested for loop.
public class Main{ public static void main(String args[]){ int mtr1[][] = {{2,7}, {9,2}}; int mtr2[][] = {{6,3}, {5,1}}; int add[][] = new int[2][2]; // Nested for loop for (int i= 0 ; i < 2 ; i++ ){ for (int j= 0 ; j < 2 ;j++ ){ add[i][j] = mtr1[i][j] + mtr2[i][j]; // Performing addition } } System.out.println("Sum of given two matrices ="); // Nested for loop to print the resulted matrix for (int i= 0 ; i < 2 ; i++ ){ for (int j= 0 ; j < 2 ;j++ ){ System.out.print(add[i][j]+"\t"); } System.out.println(); } } }
Output
Sum of given two matrices = 8 10 14 3
In the above code, we have taken two matrices ‘mtr1’ and ‘mtr2’ of size 2 rows and 2 columns. The first two for loops will run 4 times and during each iteration values of ‘mtr1’ and ‘mtr2’ will get stored in third matrix ‘add’. The last two for loops will print the result on the screen.
Nested if
Syntax
if(conditional expression){ // code to be executed if(conditional expression){ // code to be executed } }
Example
public class Main { public static void main(String []args){ String Fname= "Tutorials"; String Lname= "point"; // Nested if block if(Fname == "Tutorials") { if(Lname == "point") { System.out.println(Fname + Lname); } else { System.out.println("Last name not matched"); } } else { System.out.println("Not able to fetch info!"); } } }
Output
Tutorialspoint
In the above code, we have declared and initialized two string variables. We have taken two if condtitions one inside another. In the first if block we have checked whether the first name is equal to “Tutorials” or not. In the second block, we have checked whether the last name is equal to “point” or not. If any of the conditions becomes false then else block will get executed. In this case, the both condition are true therefore we are getting concatenated result.
Nested While loop
Syntax
while (conditional expression) { // code will be executed till conditional expression is true while (conditional expression) { // code will be executed till conditional expression is true increment/decrement expression; // to increment/decrement loop variable } increment/decrement expression; // to increment/decrement loop variable }
Example
The following program will perform addition of two matrices using while loop.
public class Main{ public static void main(String args[]){ int mtr1[][] = {{2,7},{9,2}}; int mtr2[][] = {{6,3},{5,1}}; int add[][] = new int[2][2]; int i=0; // Nested while loop to perform addition while(i<2){ int j=0; while(j<2){ add[i][j] = mtr1[i][j] + mtr2[i][j]; j++; } i++; } System.out.println("Sum of given two matrices ="); i=0; // Nested while loop to print result while(i<2){ int j=0; while(j<2){ System.out.print(add[i][j]+"\t"); j++; } System.out.println(); i++; } } }
Output
Sum of given two matrices = 8 10 14 3
In the above code, we have followed the same logic as we did in the example 1 program but here we have used nested while loop instead of for loop. Again, we have taken two matrices ‘mtr1’ and ‘mtr2’ of size 2 rows and 2 columns. The first two while loops will run 4 times and during each iteration values of ‘mtr1’ and ‘mtr2’ will get stored in third matrix ‘add’. The last two while loops will print the result on the screen.
Conclusion
Loop within another loop is called as nested loop. We have understood the nested for loops, nested if block and nested while loop with examples. These loops are used when we have to perform operations on 2-D array and matrices. We use them in pattern-based problems also.