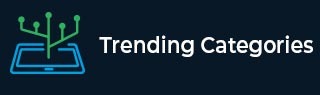
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java log10() with example
The java.lang.Math.log10(double a) returns the base 10 logarithm of a double value. Special cases −
If the argument is NaN or less than zero, then the result is NaN.
If the argument is positive infinity, then the result is positive infinity.
If the argument is positive zero or negative zero, then the result is negative infinity.
If the argument is equal to 10n for integer n, then the result is n.
Example
Following is an example to implement the log10() method in Java −
import java.lang.*; public class MathDemo { public static void main(String[] args) { double x = 56567.5; double y = 100; // get the base 10 logarithm for x System.out.println("Math.log10(" + x + ")=" + Math.log10(x)); // get the base 10 logarithm for y System.out.println("Math.log10(" + y + ")=" + Math.log10(y)); } }
Output
Math.log10(56567.5)=4.752566985524987 Math.log10(100.0)=2.0
Let us see another example −
Example
import java.lang.*; public class MathDemo { public static void main(String[] args) { double x = -30; double y = 1.0 / 0; ; // get the base 10 logarithm for x System.out.println("Math.log10(" + x + ")=" + Math.log10(x)); // get the base 10 logarithm for y System.out.println("Math.log10(" + y + ")=" + Math.log10(y)); } }
Output
Math.log10(-30.0)=NaN Math.log10(Infinity)=Infinity
Advertisements