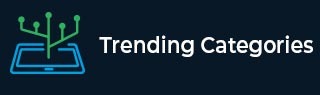
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Java 8 Streams and its operations
Streams are sequences of objects from a source, which support aggregate operations. These were introduced in Java 8.
With Java 8, Collection interface has two methods to generate a Stream.
stream() − Returns a sequential stream considering collection as its source.
parallelStream() − Returns a parallel Stream considering collection as its source.
Some operations on streams are −
sorted − The sorted method is use to sort the stream lexicographically or in ascending order
List id = Arrays.asList("Objects","Classes","Interfaces"); List output = id.stream().sorted().collect(Collectors.toList());
map − The map method maps the elements in the collection to other objects according to the Predicate passed as a parameter
List list1= Arrays.asList(1,3,5,7); List finalList = list1.stream().map(a -> a * a * a).collect(Collectors.toList());
filter − The filter method is used to select elements according to the Predicate parameter passed
List id = Arrays.asList(“Classes","Methods","Members"); List output = id.stream().filter(s -> s.startsWith("M")).collect(Collectors.toList());
The three operations above are intermediate operations. Now let us have a look at the terminal operations
collect − The collect method returns the outcome of the intermediate operations
List id = Arrays.asList(“Classes","Methods","Members"); List output = id.stream().filter(s -> s.startsWith("M")).collect(Collectors.toList());
forEach − This method iterates through every element in the stream
List list1 = Arrays.asList(1,3,5,7); List finalList = list1.stream().map(a ->a * a * a).forEach(b->System.out.println(b));
Let us see an example which illustrates the use of Stream −
Example
import java.util.*; import java.util.stream.*; public class Example { public static void main(String args[]) { List<Integer> list1 = Arrays.asList(11,22,44,21); //creating an integer list List<String> id = Arrays.asList("Objects","Classes","Methods"); //creating a String list // map method List<Integer> answer = list1.stream().map(x -> x*x).collect(Collectors.toList()); System.out.println(answer); // filter method List<String> output = id.stream().filter(x->x.startsWith("M")).collect(Collectors.toList()); System.out.println(output); } }
Output
[121, 484, 1936, 441] [Methods]