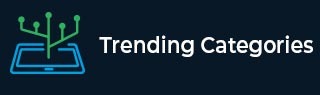
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Iterate through a LinkedList in reverse direction using a ListIterator in Java
A ListIterator can be used to traverse the elements in the forward direction as well as the reverse direction in a LinkedList. The method hasPrevious( ) in ListIterator returns true if there are more elements in the LinkedList while traversing in the reverse direction and false otherwise. The method previous( ) returns the previous element in the LinkedList and reduces the cursor position backward.
A program that demonstrates this is given as follows.
Example
import java.util.LinkedList; import java.util.List; import java.util.ListIterator; public class Demo { public static void main(String[] args) { List l = new LinkedList(); l.add("John"); l.add("Sara"); l.add("Susan"); l.add("Betty"); l.add("Nathan"); ListIterator i = l.listIterator(l.size()); System.out.println("The LinkedList elements in the reverse direction are: "); while (i.hasPrevious()) { System.out.println(i.previous()); } } }
Output
The output of the above program is as follows
The LinkedList elements in the reverse direction are: Nathan Betty Susan Sara John
Now let us understand the above program.
The LinkedList is created and LinkedList.add() is used to add the elements to the LinkedList. A code snippet which demonstrates this is as follows
List l = new LinkedList(); l.add("John"); l.add("Sara"); l.add("Susan"); l.add("Betty"); l.add("Nathan");
Then the ListIterator interface is used to display the LinkedList elements in the reverse direction. A code snippet which demonstrates this is as follows
ListIterator i = l.listIterator(l.size()); System.out.println("The LinkedList elements in the reverse direction are: "); while (i.hasPrevious()) { System.out.println(i.previous()); }