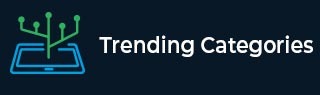
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
isfinite() function in C++
In this article we will be discussing the working, syntax and examples of isfinite() function in C++.
isfinite() is an inbuilt function in C++ which comes under header file. isfinite() function used to check and return whether the given number is finite or not, a finite number is any floating number which is neither infinite nor NaN (Not a number).
Syntax
bool isfinite(float n);
or
bool isfinite(double n);
or
bool isfinite(long double n);
This function include only 1 parameter n which is the value we have to check for whether it's finite or not.
Return value
The function returns boolean value, 0(false) if the number is not finite and 1(true) if the number is finite.
Example
#include <iostream> #include <cmath> using namespace std; int main() { float a = 10.0, b = 0.1, c = 0.0; isfinite(a/b)?cout<<"\nThe result of a/b is finite":cout<<"\nThe result of a/b is not finite"; isfinite(a/c)?cout<<"\nThe result of a/c is finite":cout<<"\nThe result of a/c is not finite"; }
Output
If we run the above code it will generate the following output −
The result of a/b is finite The result of a/c is not finite
Example
#include <iostream> #include <cmath> using namespace std; int main() { float c = 0.0, d = -1.0; //check the number is infinte or finite isfinite(c)?cout<<"\nFinite number":cout<<"\nNot a finite number"; cout<<isfinite(sqrt(d)); //Result will be -NAN }
Output
If we run the above code it will generate the following output −
Finite number 0
Note − square root of -1.0 will return nan
Advertisements