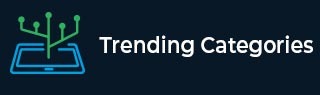
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Is there an equivalent of C’s “?:” ternary operator in Python?
Yes, we can work around C Language’s ternary operator in Python as well i.e. a similar way do exist. Let us first see an example of C Language’s ternary operator −
Example
#include <stdio.h> int main() { int x = 10; int y; y = (x == 1) ? 20: 30; printf( "Value of y = %d\n", y ); y = (x == 10) ? 20: 30; printf( "Value of y = %d\n", y ); }
Output
Value of y = 30 Value of y = 20
There are many operators in Python: Arithmetic, Assignment, Logical, Bitwise, Membership, Identity, etc. The task accomplished above by ternary in C language can be achieved by a logical operator.
Before Python 2.5, programmers worked around the following logical operator syntax to get the same work done. However this is considered incorrect and usage as it fails when on_safe is having a false Boolean −
[expression] and [on_true] or [on_false]
Therefore, after Python 2.5 the following form of ternary operator introduced to ease the task of programmers.
Syntax
Let us see the syntax −
[on_true] if [expression] else [on_false]
Example
Let us now see an example of implementing ternary operator in Python −
x = 20 y = 10 res = x if x < y else y print(res)
Output
10
Example
Here’s an example −
x = 50 y = 10 res = x if x > y else y print(res)
Output
50