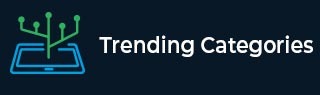
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Increment Negative and Decrement Positive Numbers by 1 in an Array in Java
In Java, Array is an object. It is a non-primitive data type which stores values of similar data type.
As per the problem statement we have to increment all negative numbers by 1 and decrement all positive numbers by 1.
Let’s explore the article to see how it can be done by using Java programming language.
To Show You Some Instances
Instance-1
Suppose the original array is {12, 21, -31, -14, 56, 16, 17, -18, 9}
After updating the array element are: -30 -17 -13 8 11 15 16 20 55 (sorted)
Instance-2
Suppose the original array is {19, 11, -21, 14, 6, -16, 17, -18, 8}
After updating the array element are: -30 -17 -13 8 11 15 16 20 55 (sorted)
Instance-3
Suppose the original array is {1, 5, -3, 4, -5, 16, 17, -12, -9}
After updating the array element are: -11 -8 -4 -2 0 3 4 15 16 (sorted)
Algorithm
Step 1 − Declare and initialize an integer array.
Step 2 − Sort array in ascending order
Step 3 − Initialize for loop to print original array elements
Step 4 − Initialize another for loop to print updated array elements by adding 1 to negative array element and subtracting 1 from positive array elements.
Step 5 − Print the elements of the array.
Syntax
To get the length of an array (number of elements in that array), there is an inbuilt property of array i.e length.
Below refers to the syntax of it −
array.length
Where, ‘array’ refers to the array reference.
Multiple Approaches
We have provided the solution in different approaches.
By Using Static Initialization of Array
By Using User Defined Method
Let’s see the program along with its output one by one.
Approach-1: By Using Static Initialization of Array
Example
In this approach, array elements will be initialized in the program. Then as per the algorithm increment all negative numbers by 1 and decrement all positive numbers by 1.
import java.util.*; public class Main { //main method public static void main(String[] args){ //Declare and initialize the array elements int[] arr = { 1, 5, -3, 4, -5, 16, 17, -12, -9 }; //sorting array in ascending order System.out.print("Original array after sorting them in ascending order: "); System.out.print("\n"); //for each loop to print original array elements for (int e : arr) System.out.print(e + " "); System.out.print("\n"); System.out.print("Array after incrementing negative and decrementing positive numbers by 1: "); System.out.print("\n"); //for loop to print array updated elements for (int e : arr){ if(e < 0){ e = e + 1; System.out.print(e + " "); } else { e = e - 1; System.out.print(e + " "); } } } }
Output
Original array after sorting them in ascending order: 1 5 -3 4 -5 16 17 -12 -9 Array after incrementing negative and decrementing positive numbers by 1: 0 4 -2 3 -4 15 16 -11 -8
Approach-2: By Using User Defined Method
Example
In this approach, array elements will be initialized in the program. Then call a user defined method by passing the array as parameter and inside method as per the algorithm increment all negative numbers by 1 and decrement all positive numbers by 1.
import java.util.*; public class Main{ //main method public static void main(String[] args){ //Declare and initialize the array elements int[] arr = { 19, 11, -21, 14, 6, -16, 17, -18, 8 }; //calling user defined method func(arr); } //user defined method public static void func(int arr[]){ //sorting array in ascending order Arrays.sort(arr); System.out.print("Original array after sorting them in ascending order: "); System.out.print("\n"); //for each loop to print original array elements for (int e : arr) System.out.print(e + " "); System.out.print("\n"); System.out.print("Array after incrementing negative and decrementing positive numbers by 1: "); System.out.print("\n"); //for loop to print array updated elements for (int e : arr){ if(e < 0){ e = e + 1; System.out.print(e + " "); } else { e = e - 1; System.out.print(e + " "); } } } }
Output
Original array after sorting them in ascending order: -21 -18 -16 6 8 11 14 17 19 Array after incrementing negative and decrementing positive numbers by 1: -20 -17 -15 5 7 10 13 16 18
In this article, we explored different approaches to increment all negative numbers by 1 and decrement all positive numbers by 1 in an array by using Java programming language.