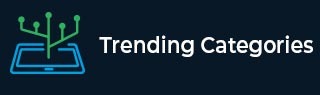
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Implementing insertion sort to sort array of numbers in increasing order using JavaScript
The art of sorting arrays is of paramount importance in the realm of programming, as it allows for efficient organization and manipulation of data. When it comes to implementing a reliable sorting algorithm, insertion sort emerges as a versatile and effective option. In this article, we delve into the intricate world of JavaScript to explore the process of implementing insertion sort to arrange an array of numbers in increasing order. By comprehending the underlying mechanics of this algorithm and harnessing the power of JavaScript's capabilities, developers can unlock the potential to efficiently sort and organize numerical data, enhancing the performance and usability of their applications.
Problem Statement
The challenge at hand pertains to the task of implementing the insertion sort algorithm utilizing JavaScript for the purpose of arranging an array of numbers in ascending order. The primary objective is to devise a program that intelligently rearranges the elements of the given array, ensuring that each subsequent element is placed in its proper position relative to the preceding elements, based on their numerical values. To exemplify, suppose we are provided with an array
[9, 2, 7, 4, 1]
Upon executing the insertion sort algorithm, the expected outcome would be an array that adheres to the increasingly ordered sequence, such as
[1, 2, 4, 7, 9]
Approach
In this article, we are going to see a number of different ways to solve the above problem statement in JavaScript −
Basic Insertion Sort
Binary Insertion Sort
Recursive Insertion Sort
Method 1: Basic Insertion Sort
The basic insertion sort algorithm maintains a sorted subarray within the array. Starting from the second element, each element is compared with the previous elements in the subarray and shifted to the right if smaller. This process continues until the correct position is found, and the element is inserted. This process is repeated for all elements, resulting in a fully sorted array.
Example
The insertionSort function takes an array arr as input and returns the sorted array using the insertion sort algorithm. It iterates from the second element, storing it in the current variable. A while loop compares the current element with previous elements in the sorted subarray, shifting larger elements to the right. The loop continues until it reaches the beginning of the array or finds a smaller element. The current element is then inserted at the correct position in the sorted subarray. This process is repeated for all elements, resulting in a sorted array.
function insertionSort(arr) { for (let i = 1; i < arr.length; i++) { let current = arr[i]; let j = i - 1; while (j >= 0 && arr[j] > current) { arr[j + 1] = arr[j]; j--; } arr[j + 1] = current; } return arr; } const arr = [9, 2, 7, 4, 1]; console.log(insertionSort(arr));
Output
The following is the console output −
[ 1, 2, 4, 7, 9 ]
Method 2: Binary Insertion Sort
The binary insertion sort algorithm enhances the efficiency of the basic insertion sort by utilizing binary search within a sorted subarray to determine the correct position for each element. Instead of linearly searching, a binary search is performed by comparing the current element with the middle element of the subarray, adjusting the search boundaries accordingly. Once the insertion point is determined, elements to the right are shifted to make room, and the current element is inserted. This process is repeated for all elements, resulting in a fully sorted array.
Example
The binaryInsertionSort function takes an array arr and returns the sorted array using the binary insertion sort algorithm. It iterates from the second element, assuming the first element is sorted. The current element is stored in the current variable. The algorithm performs a binary search within the sorted subarray to find the correct position for the current element by comparing it with the middle element and adjusting the search boundaries. Once the position is found, the algorithm shifts the elements to the right and inserts the current element at the correct position. This process is repeated for all elements, resulting in a sorted array.
function binaryInsertionSort(arr) { for (let i = 1; i < arr.length; i++) { let current = arr[i]; let left = 0; let right = i - 1; while (left <= right) { let mid = Math.floor((left + right) / 2); if (current < arr[mid]) { right = mid - 1; } else { left = mid + 1; } } for (let j = i - 1; j >= left; j--) { arr[j + 1] = arr[j]; } arr[left] = current; } return arr; } const arr = [9, 2, 7, 4, 1]; console.log(binaryInsertionSort(arr));
Output
The following is the console output −
[ 1, 2, 4, 7, 9 ]
Method 3: Recursive Insertion Sort
The recursive insertion sort algorithm is a recursive version of the insertion sort, using recursion to sort the array. For subarrays with a size of 1 or less, it considers them already sorted. For larger subarrays, it recursively calls itself to sort the subarray without the last element. After the recursive call returns and the subarray is sorted, the algorithm places the last element in its correct position within the sorted subarray. This is achieved by comparing the last element with the elements in the sorted subarray and shifting them to the right if necessary. The process repeats until all elements are inserted at their correct positions, resulting in a fully sorted array.
Example
The recursiveInsertionSort function recursively applies the insertion sort algorithm to an input array. It checks if the array is already sorted and returns it if so. Otherwise, it recursively calls itself on a subarray of size n - 1. After the recursive call, the function compares the last element with the elements in the sorted subarray using a while loop. If an element is larger, it shifts it to the right. This process continues until the loop reaches the beginning of the array or finds a smaller element. Finally, the last element is inserted at the correct position. This process is repeated for all elements, resulting in a sorted array.
function recursiveInsertionSort(arr, n = arr.length) { if (n <= 1) return arr; recursiveInsertionSort(arr, n - 1); let last = arr[n - 1]; let j = n - 2; while (j >= 0 && arr[j] > last) { arr[j + 1] = arr[j]; j--; } arr[j + 1] = last; return arr; } const arr = [9, 2, 7, 4, 1]; console.log(recursiveInsertionSort(arr));
Output
The following is the console output −
[ 1, 2, 4, 7, 9 ]
Conclusion
In finality, the implementation of insertion sort algorithm to arrange an array of numbers in ascending order using JavaScript is an astute choice for developers seeking a proficient sorting method. By iteratively placing elements in their appropriate positions, this algorithm exhibits a discerning approach to organizing numerical data. While insertion sort may not be as widely acclaimed as other sorting techniques, its efficiency and simplicity make it an invaluable tool in certain scenarios. Employing this algorithm in JavaScript empowers developers to wield a lesser-known yet potent tool in their coding arsenal, resulting in streamlined and ordered arrays. In conclusion, harnessing the power of insertion sort algorithm in JavaScript promises a quixotic endeavor for those seeking precision and elegance in their array sorting endeavors.