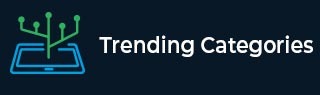
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Implementing Heap Sort using vanilla JavaScript
Heap Sort is basically a comparison-based sorting algorithm. It can be thought of as an improved selection sort − like that algorithm, it divides its input into a sorted and an unsorted region, and it interactively shrinks the unsorted region by extracting the target (largest or smallest) element and moving that to the sorted region.
Example
The code for this will be −
const constructHeap = (arr, ind) => { let left = 2 * ind + 1; let right = 2 * ind + 2; let max = ind; if (left < len && arr[left] > arr[max]) { max = left; } if (right < len && arr[right] > arr[max]) { max = right; } if (max != ind) { swap(arr, ind, max); constructHeap(arr, max); } } function swap(arr, index_A, index_B) { let temp = arr[index_A]; arr[index_A] = arr[index_B]; arr[index_B] = temp; } function heapSort(arr) { len = arr.length; for (let ind = Math.floor(len / 2); ind >= 0; ind −= 1) { constructHeap(arr, ind); } for (ind = arr.length − 1; ind > 0; ind−−) { swap(arr, 0, ind); len−−; constructHeap(arr, 0); } } const arr = [3, 0, 2, 5, −1, 4, 1]; heapSort(arr); console.log(arr); var len;
Output
And the output in the console will be −
[ −1, 0, 1, 2, 3, 4, 5 ]
Advertisements