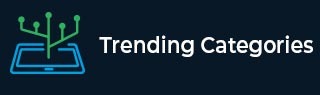
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Implementing circular queue ring buffer in JavaScript
Circular Queue
The circular queue is a linear data structure in which the operations are performed based on FIFO (First In First Out) principle and the last position is connected back to the first position to make a circle. It is also called "Ring Buffer".
One of the benefits of the circular queue is that we can make use of the spaces in front of the queue. In a normal queue, once the queue becomes full, we cannot insert the next element even if there is a space in front of the queue. But using the circular queue, we can use the space to store new values.
Problem
We are required to design our implementation of the circular queue in JavaScript that can support the following operations −
MyCircularQueue(k) − Constructor, set the size of the queue to be k.
Front() − Get the front item from the queue. If the queue is empty, return -1.
Rear() − Get the last item from the queue. If the queue is empty, return -1.
enQueue(value) − Insert an element into the circular queue. Return true if the operation is successful.
deQueue() − Delete an element from the circular queue. Return true if the operation is successful.
isEmpty() − Checks whether the circular queue is empty or not.
isFull() − Checks whether the circular queue is full or not.
Example
Following is the code −
const CircularQueue = function(k) { this.size = k this.queue = [] this.start1 = 0 this.end1 = 0 this.start2 = 0 this.end2 = 0 } CircularQueue.prototype.enQueue = function(value) { if(this.isFull()) { return false } if(this.end2 <= this.size - 1) { this.queue[this.end2++] = value } else { this.queue[this.end1++] = value } return true } CircularQueue.prototype.deQueue = function() { if(this.isEmpty()) { return false } if(this.queue[this.start2] !== undefined) { this.queue[this.start2++] = undefined } else { this.queue[this.start1++] = undefined } return true } CircularQueue.prototype.Front = function() { if(this.isEmpty()) { return -1 } return this.queue[this.start2] === undefined ? this.queue[this.start1] : this.queue[this.start2] } CircularQueue.prototype.Rear = function() { if(this.isEmpty()) { return -1 } return this.queue[this.end1 - 1] === undefined ? this.queue[this.end2 - 1] : this.queue[this.end1 - 1] } CircularQueue.prototype.isEmpty = function() { if(this.end2 - this.start2 + this.end1 - this.start1 <= 0) { return true } return false } CircularQueue.prototype.isFull = function() { if(this.end2 - this.start2 + this.end1 - this.start1 >= this.size) { return true } return false } const queue = new CircularQueue(2); console.log(queue.enQueue(1)); console.log(queue.enQueue(2)); console.log(queue.enQueue(3)); console.log(queue.Rear()); console.log(queue.isFull()); console.log(queue.deQueue()); console.log(queue.enQueue(3)); console.log(queue.Rear());
Output
true true false 2 true true true 3