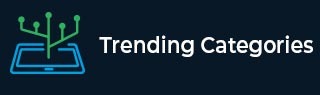
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
HTML DOM childNodes Property
The HTML DOM childNodes property returns a collection of node’s child nodes in the form of a NodeList object. The nodes are sorted and are in the same order as they appear in the HTML document. These nodes can be accessed by index number which starts from 0. It is a read-only property. Remember, white spaces and comments are also considered as nodes.
Syntax
Following is the syntax for childNodes property −
elementNodeReference.childNodes;
Example
Let us see an example for the HTML DOM childNodes property −
<!DOCTYPE html> <html> <head> <style> div { border: 1px solid blue; margin: 7px; } </style> </head> <body> <p>Click the button below to find the div element childnodes.</p> <button onclick="countNodes()">COUNT</button> <div id="DIV1"> <p>First p element </p> <p>Second p element</p> </div> <p id="Sample"></p> <script> function countNodes() { var x = document.getElementById("DIV1").childNodes.length; document.getElementById("Sample").innerHTML = "The div element has "+x+" child nodes"; } </script> </body> </html>
Output
This will produce the following output −
On clicking the COUNT button −
In the above example −
We have created a <div> element with id “DIV1” and it has two paragraphs inside it. We have added a color border and some margin to distinguish it from other elements. Important thing here is that there are three enter key pressed to get each element on its own line which creates total three whitespaces in out <div> tag.
div { border: 1px solid blue; margin: 7px; } <div id="DIV1"> <p>First p element </p> <p>Second p element</p> </div>
We have then created a button COUNT that will execute the countNodes() method when clicked upon by user.
<button onclick="countNodes()">COUNT</button>
The countNodes() function gets the element which has id equals “DIV1” which in our case is the <div> element and gets its childnodes.length property value and assigns it to the variable x.
Since there are three whitespaces and two <p> elements inside the <div>tag the childnodes.length return 5. The returned value is then displayed in the paragraph with id “Sample” using the innerHTML() method.
function countNodes() { var x = document.getElementById("DIV1").childNodes.length; document.getElementById("Sample").innerHTML = "The div element has "+x+" child nodes"; }