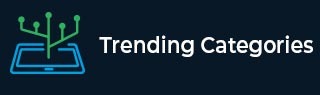
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to write a class inside an interface in Java?
Defining a class within an interface is allowed in Java. If the methods of an interface accept a class as an argument and the class is not used elsewhere, in such cases we can define a class inside an interface.
Example
In the following example we have an interface with name CarRentalServices and this interface has two methods that accepts an object of the class Car as an argument. Within this interface we have the class Car.
interface CarRentalServices { void lendCar(Car c); void collectCar(Car c); public class Car{ int carId; String carModel; int issueDate; int returnDate; } } public class InterfaceSample implements CarRentalServices { public void lendCar(Car c) { System.out.println("Car Issued"); } public void collectCar(Car c) { System.out.println("Car Retrieved"); } public static void main(String args[]){ InterfaceSample obj = new InterfaceSample(); obj.lendCar(new CarRentalServices.Car()); obj.collectCar(new CarRentalServices.Car()); } }
Output
Car Issued Car Retrieved
Advertisements