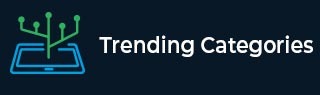
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to use the series.isin() method to check values in a series?
The Pandas series.isin() function is used to check whether the requested values are contained in the given Series object or not. It will return a boolean series object showing whether each element in the series matches the elements in the past sequence to the isin() method.
The boolean value True represents the matched elements in series that are specified in the input sequence of the isin() method, and not matched elements are represented with False.
The isin() method expects only a sequence of values and not a Series of sequences or a direct value. This means, it allows vectorization on keys but not on values.
It will raise TypeError, if any other data passed, only list-like objects are allowed to be passed to isin() method.
Example 1
We will use the Series.isin() function to check whether the passed values are available in the series object or not.
# importing pandas package import pandas as pd #creating pandas Series series = pd.Series([7, 2, 6, 2, 5, 4, 1, 2, 3, 8]) print(series) # Apply isin() function to check for the specified values result = series.isin([2]) print("Output:") print(result)
Output
The output is as follows −
0 7 1 2 2 6 3 2 4 5 5 4 6 1 7 2 8 3 9 8 dtype: int64 Output: 0 False 1 True 2 False 3 True 4 False 5 False 6 False 7 True 8 False 9 False dtype: bool
As we can see in the output block, the Series.isin() method has returned a new series object with boolean values. True indicates the values at that particular instance are matched to the given sequence. False indicates Reverse.
Example 2
Here we will see how to check multiple values at a time by passing a list of values to the isin() method.
# importing pandas package import pandas as pd #creating pandas Series series = pd.Series(['A', 'B', 'C', 'A', 'D', 'E', 'B'], index=[1, 2, 3, 4, 5, 6, 7]) print("Series object:") print(series) # Apply isin() function to check for the specified values result = series.isin(['A', 'B']) print("Output:") print(result)
Output
The output is given below −
Series object: 1 A 2 B 3 C 4 A 5 D 6 E 7 B dtype: object Output: 1 True 2 True 3 False 4 True 5 False 6 False 7 True dtype: bool
In the above output block, We can observe that the values at index 1, 2, 4, and 7 are matched to the given sequence of values, while the remaining are not.