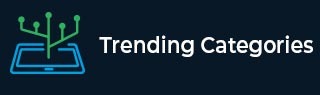
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to use regular expression in Java to pattern match?
A regular expression is a string of characters that defines/forms a pattern to search an input text. A regular expression may contain one or more characters, using a regular expression you can search or replace a string.
Java provides the java.util.regex package for pattern matching with regular expressions. The pattern class of this package is a compiled representation of a regular expression. To match a regular expression with a String this class provides two methods namely −
- compile(): This method accepts a String representing a regular expression and returns an object of the Pattern object.
- matcher(): This method accepts a String value and creates a matcher object which matches the given String to the pattern represented by the current pattern object.
Example
Following Java program accepts a string from the user, verifies whether it contains alphabet (both cases), It also accepts digits.
import java.util.Scanner; import java.util.regex.Matcher; import java.util.regex.Pattern; public class Test { public static void main( String args[] ) { String regex = "[a-zA-Z][0-9]?"; Scanner sc = new Scanner(System.in); System.out.println("Enter an input string: "); String input = sc.nextLine(); //Creating a Pattern object Pattern p = Pattern.compile(regex); //Creating a Matcher object Matcher m = p.matcher(input); if(m.find()) { System.out.println("Match occurred"); }else { System.out.println("Match not occurred"); } } }
Output 1
Enter an input string: sample text Match occurred
Output 2
Enter an input string: sample text 34 56 Match occurred
Advertisements