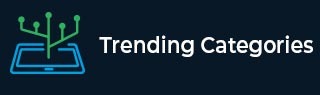
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to use protractor to wait for new tab to be created?
Popular end-to-end testing framework Protractor performs tests against a web application in a real browser, mimicking user interactions. It is used with Angular and AngularJS apps. A new tab or window may open when a user hits a link or button in an online application. This is a typical scenario. It is crucial in these circumstances to wait for the new tab to be formed before making any additional changes to it.
We can use the browser to wait for Protractor to open a new tab in conjunction with the browser's wait() feature. The method getAllWindowHandles() A list of all the window handles in the current session is returned by the browser.getAllWindowHandles() function. We can utilize this function to see if a new tab has been opened by watching for the number of window handles to rise.
The browser.wait() method can delay running the test until a specific requirement has been met. In this instance, we can watch for the number of window handles to rise, signifying the opening of a new tab. The wait function will throw an error if the condition is not satisfied in the allotted time.
This method allows us to ensure that our tests wait for the creation of new tabs before doing any further actions, making them accurate and dependable.
Steps to use Protractor to Wait for a new tab to be Created
These methods will help us ensure that our Protractor tests wait for the creation of new tabs before taking any further actions, making them accurate and dependable.
Step 1 - Recognize the component, such as a button or link, that causes a new tab to open.
Step 2 - Locate the element using the element() function and simulate a click event using the click() method.
Step 3 - Use the browser to wait for the new tab to open.wait() function. Use the browser within the browser.wait() function. To obtain a list of all the window handles in the current session, use the getAllWindowHandles() function.
Step 4 - Examine the window handles list's length with the anticipated number of window handles. A new tab has been opened if the length of the window handles list has risen.
Step 5 - Once the new tab has been formed, transfer the attention to it and take any additional steps using the browser.switchTo().window() function.
Step 6 - The browser.wait() function will generate an error, and the test will fail if the new tab does not open within the allotted time.
Using Browser.wait() Function
Protractor uses the browser.wait() function to delay starting the test until a specific requirement has been satisfied. It accepts as its first argument a function that returns a promise or a boolean value and as its second argument a millisecond timeout. The condition will be polled repeatedly by the function until it is satisfied or the timeout is reached, which will throw an error. This function can be used to wait for asynchronous events like loading a page or opening a new tab.
Example
In this example, we will use protractor to wait for a new tab to be created. Firstly, we need to configure the conf.js file with the required information, and then we need to do coding in the test-file1.js file, which is the test case file in this example. In that file, we describe the steps and code each step for automation, and finally, we declare the index.html file to be tested. We have a button in this HTML file, and by clicking on that, a new tab pops up.
conf.js
// An example configuration file. exports.config = { directConnect: true, // Capabilities to be passed to the webdriver instance. capabilities: { 'browserName': 'chrome', }, // Framework to use. Jasmine is recommended. framework: 'jasmine', // Spec patterns are relative to the current working directory when // protractor is called. specs: ['test-file1.js'], SELENIUM_PROMISE_MANAGER: false, // Options to be passed to Jasmine. jasmineNodeOpts: { defaultTimeoutInterval: 30000 } };
test-file1.js
describe("Protractor Test App", function () { it("Test 1", async function () { var EC = protractor.ExpectedConditions; // Disable the wait for an Angular render update await browser.waitForAngularEnabled(false); // Get the HTML file that must be checked await browser.get("http://127.0.0.1:5500/index.html"); // To open a new tab, click on the element var myBtnElement = element(by.id("myBtn")); await myBtnElement.click(); // Waits for the creation of a new tab await browser.wait( async function () { var myHandler = await browser.driver.getAllWindowHandles(); await browser.switchTo() .window(await myHandler.pop()); var url = await browser.getCurrentUrl(); return await url.match( "http://127.0.0.1:5500/index2.html"); }, 10000, "Too much time to load!" ); }); });
index.html
<html> <body> <h4>Use <i>protractor</i> to wait for new tab to be created</h4> <p>Click the below button to open a new tab</p> <button id="myBtn" onclick="openNewTab()">Open New Tab</button> <script> function openNewTab() { window.open('http://127.0.0.1:5500/index2.html', '_blank') } </script> </body> </html>
Output
Protractor is a handy tool to do automated testing. This is one of its use cases of it.