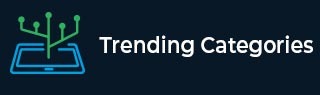
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to use intermediate stream operations in JShell in Java 9?
JShell is a tool introduced in Java 9, and it accepts simple statements like expressions, variables, methods, classes, etc.. as input and produces immediate results.
A Stream is a sequence of values. An intermediate stream operation is an operation that takes a stream. For instance, it can be applied to a lambda expression and produces another stream of elements as its result.
The most popular intermediate stream operations are mentioned below:
- 1) sorted(): This method preserves the elements of the consumed stream as a result but also puts them in natural sorted order.
- 2) distinct(): This method returns a stream retaining only unique elements of the input stream, and it can maintain the relative order of retained elements.
- 3) filter(): This method can be used to filter stream elements based on some logic.
- 4) map(): This method applies lambda expression to compute new results from input stream elements. Then, it returns a stream of these results as output.
In the below code snippet, we can implement different intermediate stream operations in the JShell tool.
Snippet
jshell> List<Integer> numbers = List.of(3, 10, 23, 200, 77, 9, 32); numbers ==> [3, 10, 23, 200, 77, 9, 32] jshell> numbers.stream().sorted().forEach(elem -> System.out.println(elem)); 3 9 10 23 32 77 200 jshell> List<Integer> numbers = List.of(3, 5, 54, 280, 5, 9, 40); numbers ==> [3, 5, 54, 280, 5, 9, 40] jshell> numbers.stream().distinct().forEach(elem -> System.out.println(elem)); 3 5 54 280 9 40 jshell> numbers.stream().distinct().sorted().forEach(elem -> System.out.println(elem)); 3 5 9 40 54 280 jshell> numbers.stream().distinct().map(num -> num * num).forEach(elem -> System.out.println(elem)); 9 25 2916 78400 81 1600
Advertisements