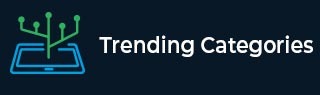
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to upload files using jQuery Dropzone Plugin?
Uploading files on a website can be a challenging task, especially when you have to handle different types of files, such as images, videos, and documents. Fortunately, jQuery Dropzone Plugin makes it easy to handle file uploads with just a few lines of code. There are many more plugins available for uploading the files like Fine-Uploader, JQuery File upload, Uploadify, etc.
In this article, we will learn how to upload files using the jQuery Dropzone plugin. We will go through the complete process of using the jQuery Dropzone Plugin to upload files on the website.
What is jQuery Dropzone Plugin?
jQuery Dropzone Plugin is a powerful and easy-to-use plugin that allows users to upload files to a server using drag-and-drop functionality. The plugin comes with a lot of customization options and features that make it easy to handle file uploads in different scenarios. Some of the features of jQuery Dropzone Plugin include −
Support for different types of files
Ability to upload multiple files at once
Automatic file size detection and validation
Drag and drop file upload
Customizable upload progress indicators
Image preview before upload
Now that we know what jQuery Dropzone Plugin is, let's move on to the steps required to use it in our website.
Steps to Upload Files Using jQuery Dropzone Plugin
Step 1: Download or use CDN to Include jQuery Dropzone Plugin in the Project
The first step is to download the latest version of jQuery and Dropzone Plugin from their respective websites. Or we can also use the CDN links to include these files in our project. Below are CDN links available that need to be included in the HTML.
CDN to Use
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/dropzone/5.9.2/min/dropzone.min.css"/> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dropzone/5.9.2/min/dropzone.min.js"></script>
Step 2: Add a Form to Use Dropzone Plugin
The second step is to include the latest version of jQuery and Dropzone Plugin using CDN links. Below is a syntax used to add a form element with the class "dropzone" where the uploaded files will be displayed.
<html> <head> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/dropzone/5.9.2/min/dropzone.min.css"/> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dropzone/5.9.2/min/dropzone.min.js"></script> </head> <body> <div class="container"> <div class="row"> <div class="col-md-12"> <form action="your-upload-file.php" class="dropzone"></form> </div> </div> </div> </body> </html>
Step 3: Configure the Plugin Options
The next step is to configure the options for Dropzone Plugin. We can do this by creating a new instance of Dropzone and passing the configuration options as an object.
<script> $(function() { var myFileUploadDropZone = new Dropzone(".dropzone", { url: "your-upload-file", maxFiles: 15, maxFilesize: 5, acceptedFiles: ".png, .jpg, .gif, .pdf, .doc", addRemoveLinks: true, dictDefaultMessage: "Drop your files here or click to upload", dictFallbackMessage: "Your browser does not support drag & drop feature.", dictInvalidFileType: "Your uploaded file type is not supported.", dictFileTooBig: "File is too big ({{filesize}} MB). Max filesize: {{maxFilesize}} MB.", dictResponseError: "Server responded with {{statusCode}} code.", dictCancelUpload: "Cancel Upload", dictRemoveFile: "Remove", init: function() { this.on("complete", function(file) { this.removeFile(file); }); } }); }); </script>
In this example, we have passed the following options to the Dropzone instance −
url − The URL where the uploaded files will be sent.
maxFiles − The maximum number of files that can be uploaded.
maxFilesize − The maximum size of each file in megabytes.
acceptedFiles − The types of files that can be uploaded.
addRemoveLinks − Whether or not to add a remove link for uploaded files.
dictDefaultMessage − The default message to display when there are no files uploaded.
dictFallbackMessage − The message to display when the browser does not support drag and drop file uploads.
dictInvalidFileType − The message to display when the user tries to upload a file that is not supported.
dictFileTooBig − The message to display when the user tries to upload a file that is too big.
dictResponseError − The message to display when the server responds with an error code.
dictCancelUpload − The label for the cancel upload button.
dictRemoveFile − The label for the remove file button.
We have also added an event listener to the "complete" event, which will remove the uploaded file from the interface after it has been uploaded successfully.
Step 4: Create a PHP File to Handle File Uploads
Our next step is to create a PHP file that will handle the uploaded files on the server side. Below is the syntax of a simple PHP script that will handle file uploads −
<?php $targetDir = "uploads/"; $targetFile = $targetDir . basename($_FILES["file"]["name"]); if (move_uploaded_file($_FILES["file"]["tmp_name"], $targetFile)) { echo "The file ". basename( $_FILES["file"]["name"]). " has been uploaded."; } else { echo "Sorry, there was an error uploading your file."; } ?>
Now its time to test our code we have configured the Dropzone Plugin and created the PHP file to handle file uploads, we can test the file upload functionality on our website. Simply drag and drop a file onto the Dropzone area or click on the area to browse for files. After the files are uploaded, you should see them displayed on the interface with an option to remove them. Below is a complete running example in HTML.
Example
Below is a complete example to demonstrate how to use the Dropzone plugin to upload the files.
<html> <head> <title>Example demonstrating how to upload files using jQuery Dropzone Plugin</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/dropzone/5.7.0/min/dropzone.min.css"> </head> <body> <h3>Example demonstrating how to upload files using jQuery Dropzone Plugin</h3> <form action="your-upload-file.php" class="dropzone"> <div class="fallback"> <input type="file" name="file" multiple> </div> </form> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dropzone/5.7.0/min/dropzone.min.js"></script> <script> $(function() { var myFileUploadDropZone = new Dropzone(".dropzone", { url: "your-upload-file", maxFiles: 15, maxFilesize: 5, acceptedFiles: ".png, .jpg, .gif, .pdf, .doc", addRemoveLinks: true, dictDefaultMessage: "Drop your files here or click to upload", dictFallbackMessage: "Your browser does not support drag & drop feature.", dictInvalidFileType: "Your uploaded file type is not supported.", dictFileTooBig: "File is too big ({{filesize}} MB). Max filesize: {{maxFilesize}} MB.", dictResponseError: "Server responded with {{statusCode}} code.", dictCancelUpload: "Cancel Upload", dictRemoveFile: "Remove", init: function() { this.on("complete", function(file) { this.removeFile(file); }); } }); }); </script> </body> </html>
Conclusion
jQuery Dropzone Plugin is a powerful and easy-to-use plugin for uploading files to a server using drag-and-drop functionality. In this article, we have discussed the steps required to use the jQuery Dropzone Plugin to upload files on the website using CDN.