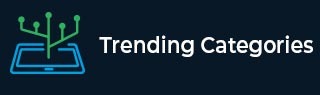
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to update parent state in ReactJS?
In this article, we are going to see how to update the parent state from a child component in a React application.
To update the parent state from the children component, either we can use additional dependencies like Redux or we can use this simple method of passing the state of the parent to the children and handling it accordingly.
Example
In this example, we will build a React application which takes the state and the method to update it from the parent component and pass it to the children component and after handling it we will pass the updated state to the parent component.
App.jsx
import React, { useLayoutEffect, useState } from 'react'; const App = () => { const [name, setName] = useState('Rahul'); return ( <div> {name} has email id of rahul@tutorialspoint.com <div> <Child name={name} change={setName} /> </div> </div> ); }; const Child = ({ name, change }) => { const [newName, setNewName] = useState(name); return ( <div> <input placeholder="Enter new name" value={newName} onChange={(e) => setNewName(e.target.value)} /> <button onClick={() => change(newName )}>Change</button> </div> ); }; export default App;
In the above example, whenever the user types in the new name, then it changes and updates the state of the child component and when clicked on the change button, then it will update the state of the parent component accordingly.
Output
This will produce the following result.