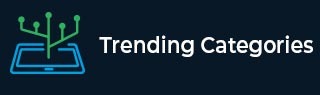
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to style the separator using CSS in JavaFX?
A separator is a horizontal or, the vertical line separating the UI elements of an application. In JavaFX the javafx.scene.control.Separator class represents a separator, to create a separator you need to instantiate this class. You can control the visual appearance of the separator using CSS.
You create an alternative style to the separator, by creating a CSS file and enabling it in your application. Once you do so, the created style will be applied to all the separators in the application.
The setStyle() method accepts the CSS in string format and applies the specified style to the separator. Using this method you can enhance the visualization of the separator as shown below −
//Setting style for the separator using CSS sep.setStyle("-fx-border-color:#D2691E; -fx-border-width:2");
Example
import javafx.application.Application; import javafx.geometry.HPos; import javafx.geometry.Insets; import javafx.geometry.Orientation; import javafx.scene.Scene; import javafx.scene.control.CheckBox; import javafx.scene.control.Label; import javafx.scene.control.Separator; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.scene.paint.Color; import javafx.scene.text.Font; import javafx.scene.text.FontPosture; import javafx.scene.text.FontWeight; import javafx.stage.Stage; public class StylingSeparator extends Application { public void start(Stage stage) { //Creating the check boxes CheckBox checkBox1 = new CheckBox("Java"); CheckBox checkBox2 = new CheckBox("Python"); CheckBox checkBox3 = new CheckBox("C++"); CheckBox checkBox4 = new CheckBox("MongoDB"); CheckBox checkBox5 = new CheckBox("Neo4J"); CheckBox checkBox6= new CheckBox("Casandra"); //Creating the label Label label = new Label("Select Technologies:"); Font font = Font.font("verdana", FontWeight.BOLD, FontPosture.REGULAR, 12); label.setFont(font); //Creating a separator Separator sep = new Separator(); sep.setOrientation(Orientation.VERTICAL); sep.setMaxHeight(75); sep.setHalignment(HPos.CENTER); //Setting style for the separator using CSS sep.setStyle("-fx-border-color:#D2691E; -fx-border-width: 2;"); //Adding the check boxes and separator to the pane VBox box1 = new VBox(5); box1.setPadding(new Insets(5, 5, 5, 50)); box1.getChildren().addAll(checkBox1, checkBox2, checkBox3); VBox box2 = new VBox(5); box2.setPadding(new Insets(5, 5, 5, 10)); box2.getChildren().addAll(checkBox4, checkBox5, checkBox6); HBox root = new HBox(box1, box2); root.getChildren().add(1,sep); //Setting the stage Scene scene = new Scene(root, 595, 130, Color.BEIGE); stage.setTitle("Separator Example"); stage.setScene(scene); stage.show(); } public static void main(String args[]){ launch(args); } }
Output
Advertisements