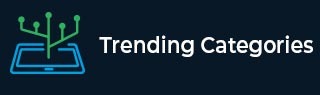
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to Sort/Order keys in JavaScript objects ?
In JavaScript, an object contains the key-value pair. Sometimes, we may require to sort the object keys. We can’t use the sort() method directly with the object as like array, as we need to sort the key-value pair of the object together rather than sorting only keys.
Below, we will learn various methods to sort the object keys in ascending and descending order.
Use the sort() method to order keys in JavaScript objects
We can use the Object.keys() method to get all keys of the object in the array. After that, we can use the array.sort() method to sort all keys, and we can create a new object from the sorted keys.
Syntax
Users can follow the syntax below to use the sort() method to sort keys in JavaScript objects.
allKeys.sort(); let temp_obj = {}; for (iterate through keys) { temp_obj[allKeys[i]] = test_obj[allKeys[i]] }
In the above syntax, first, we have sorted all keys of an object and created another object using the sorted keys.
Steps
Step 1 − Use the Object.keys() method to get all keys of the objects in the array.
Step 2 − Use the sort() method to sort all keys in ascending order by default.
Step 3 − Create a temp_obj object and initialize it with an empty object.
Step 4 − Use the for loop to iterate through all sorted keys and add key-value pair in the temp_obj object.
Step 5 – Once the iteration of for loop completes, the temp_obj object contains all keyvalue pairs of the original object in the sorted order.
Example
In the example below, when the user presses the button, it invokes the sort_obj_keys() function. After that, we created the test_obj object, which contains the key-value pairs in random order.
In the sort_obj_key() function, we get keys in the array, sort the array, add sorted keyvalue pairs in the temp_obj object. In the output users can observe that, all key-value pairs is sorted in the ascending order.
<html> <body> <h2>Sorting the <i> keys of the object in ascending order </i> in JavaScript </h2> <div id = "output"> </div> <button id = "btn"> Sort object keys </button> </body> <script> let output = document.getElementById('output'); let test_obj = { prop1: "value1", prop7: "Value7", prop4: "Value4", prop5: "Value5", prop6: "Value6", prop3: "Value3", prop2: "Value2", } function sort_obj_keys() { let allKeys = Object.keys(test_obj); allKeys.sort(); let temp_obj = {}; for (let i = 0; i < allKeys.length; i++) { temp_obj[allKeys[i]] = test_obj[allKeys[i]] } output.innerHTML += "The sorted object is <br>"; for (let key in temp_obj) { output.innerHTML += "Key - " + key + " , value - " + temp_obj[key] + " <br> "; } } let button = document.getElementById('btn'); button.addEventListener('click', () => { sort_obj_keys() }) </script> </html>
Example
In the example below, we have sorted the all object key-value pairs in the reverse order. We have first sorted the all keys of object using the sort() method. After that, we used the reverse() method to reverse the array and sort object keys in descending order.
In the output, users can observe that all key-value pairs of test_obj object are sorted in descending order.
<html> <body> <h2>Sorting the <i>keys of the object in descending order</i> in JavaScript</h2> <div id = "output"> </div> <button id = "btn" onclick = "sort_obj_keys()"> Sort object keys </button> <script> let output = document.getElementById('output'); let test_obj = { a: 10, b: 30, z: 50, x: 40, h: 21, t: 20 } function sort_obj_keys() { let allKeys = Object.keys(test_obj); // sort keys allKeys.sort(); // reverse array to sort in descending order allKeys.reverse(); let temp_obj = {}; for (let key of allKeys) { temp_obj[key] = test_obj[key] } output.innerHTML += "The sorted object is <br>"; for (let key in temp_obj) { output.innerHTML += "Key - " + key + " , value - " + temp_obj[key] + " <br> "; } } </script> </body> </html>
Use the sort() and reduce() methods to sort keys in JavaScript objects
As we have seen in the above examples, the sort() method is used to sort the array of all object keys, which we can get using the Object.keys() method.
The reduce() method reduces the sorted array of keys into a single object.
Syntax
Users can follow the syntax below to use the sort() and reduce() methods to sort the object keys.
let sorted_obj = Object.keys(house_obj) .sort().reduce((temp_obj, key) => { temp_obj[key] = house_obj[key]; return temp_obj; }, {});
In the above syntax, Object.keys() is used to get keys, sort () is to sort an array of keys, and reduce() method is used with the sorted array. The callback function of reduce() method stores the current key-value pair in the temp_obj object and returns that.
Example
In the example below, we have created the house_obj object containing the various properties of the house. After that, we used the sort() and reduce() methods to sort object keys.
The reduce() method returns the final sorted object stored in the sorted_obj variable.
<html> <body> <h2>Sorting the <i>keys of an object using sort() and reduce() methods</i> in JavaScript</h2> <div id = "output"> </div> <button id = "btn" onclick = "sort_obj_keys()"> Sort object keys </button> </body> <script> let output = document.getElementById('output'); let house_obj = { rooms: 4, color: "aqua", solar: true, window: 6, kitchen: true, bedRooms: 2, storeRoom: 1, } function sort_obj_keys() { let sorted_obj = Object.keys(house_obj) .sort().reduce((temp_obj, key) => { temp_obj[key] = house_obj[key]; return temp_obj; }, {}); output.innerHTML += "The sorted object is <br>"; for (let key in sorted_obj) { output.innerHTML += "Key - " + key + " , value - " + sorted_obj[key] + " <br> "; } } </script> </html>
In this tutorial, users learned to sort object keys in ascending and descending order. In the first approach, we used the sort() method and the for loop, and in the second approach, we used the sort() and reduce() methods.
Also, we have stored the sorted object in the temporary object variable. Still, users can delete the temporary object at the end of the code execution and initialize the original object again.