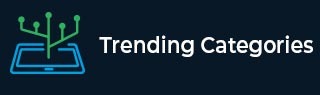
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to Sort Elements in Lexicographical Order (Dictionary Order) in Golang?
In this tutorial, we will write the Golang program to sort the elements in lexicographical order. The tutorial will include three different ways to do this thing. To do the sorting we need to compare two strings and for that, we will use the < operator it will return a boolean value. If the value on the left side is greater than the right side value lexicographically then it will return false else true.
For example,
Tutorial < Point - The operator will return true.
C++ < Golang - The operator will return false.
Algorithm
Step 1 − Create and initialize a slice of type string.
Step 2 − Running nested for loops inside which we are selecting each index from the left and comparing with the index greater than that index and storing the minimum element among the remaining elements.
Step 3 − Printing the sorted elements.
Example
In this example, we are going to sort the element in lexicographical order within the function.
package main // fmt package provides the function to print anything import "fmt" func main() { // creating a slice of string type and storing the element stringSlice := [5]string{"Tutorial", "Point", "Java", "C++", "Golang"} var temp string fmt.Println("Program to sort the element in lexicographical order within the function.") fmt.Println() fmt.Println("Elements before sorting in lexicographical order.") // printing the elements before sorting them in lexicographical order for i := 0; i < 5; i++ { fmt.Print(stringSlice[i], " ") } fmt.Println() // running the nested loops and picking one index at a time // and find the right element in lexicographical order // on that index for i := 0; i < 5; i++ { for j := i + 1; j < 5; j++ { // comparing the strings at index i and index j // if string at index i is greater in lexicographical // order than doing the swap of both elements if stringSlice[i] > stringSlice[j] { temp = stringSlice[i] stringSlice[i] = stringSlice[j] stringSlice[j] = temp } } } fmt.Println() fmt.Println("Elements after sorting in lexicographical order.") // printing the elements after sorting them in lexicographical order for i := 0; i < 5; i++ { fmt.Print(stringSlice[i], " ") } fmt.Println() }
Output
Program to sort the element in lexicographical order within the function. Elements before sorting in lexicographical order. Tutorial Point Java C++ Golang Elements after sorting in lexicographical order. C++ Golang Java Point Tutorial
Algorithm
Step 1 − Create and initialize a slice of type string.
Step 2 − Calling a function and passing the slice as an argument.
Step 3 −In the called function we are running nested for loops inside which we are selecting each index from the left and comparing with the index greater than that index and storing the minimum element among the remaining element.
Step 4 − Printing the sorted elements.
Example
In this example, we will sort the element in lexicographical order in a separate function.
package main // fmt package provides the function to print anything import "fmt" // this function has a parameter of type string slice func sortElementLexicographical(stringSlice []string) { var temp string // running the nested loops and picking one index at a time // and find the right element in lexicographical order // on that index for i := 0; i < 5; i++ { for j := i + 1; j < 5; j++ { // comparing the strings at index i and index j // if string at index i is greater in lexicographical // order than doing the swap of both elements if stringSlice[i] > stringSlice[j] { temp = stringSlice[i] stringSlice[i] = stringSlice[j] stringSlice[j] = temp } } } } func main() { // creating a slice of string type and storing the element stringSlice := [5]string{"Tutorial", "Point", "Java", "C++", "Golang"} fmt.Println("Program to sort the element in lexicographical order in the separate function.") fmt.Println() fmt.Println("Elements before sorting in lexicographical order.") // printing the elements before sorting them in lexicographical order for i := 0; i < 5; i++ { fmt.Print(stringSlice[i], " ") } fmt.Println() // calling the function by passing the stringSlice as an argument sortElementLexicographical(stringSlice[:]) fmt.Println() fmt.Println("Elements after sorting in lexicographical order.") // printing the elements after sorting them in lexicographical order for i := 0; i < 5; i++ { fmt.Print(stringSlice[i], " ") } fmt.Println() }
Output
Program to sort the element in lexicographical order in the separate function. Elements before sorting in lexicographical order. Tutorial Point Java C++ Golang Elements after sorting in lexicographical order. C++ Golang Java Point Tutorial
Algorithm
Step 1 − Create and initialize a slice of type string.
Step 2 − Calling a function from the sort package and passing the slice as an argument.
Step 3 − Printing the sorted elements.
Example
In this example, we are going to use the sort package and its function to achieve this thing.
package main // fmt package provides the function to print anything import ( "fmt" "sort" ) func main() { // creating a slice of string type and storing the element stringSlice := [5]string{"Tutorial", "Point", "Java", "C++", "Golang"} fmt.Println("Program to sort the element in lexicographical order using sort package.") fmt.Println() fmt.Println("Elements before sorting in lexicographical order.") // printing the elements before sorting them in lexicographical order for i := 0; i < 5; i++ { fmt.Print(stringSlice[i], " ") } fmt.Println() // calling the function in the sort package by passing the stringSlice as an argument sort.Strings(stringSlice[:]) fmt.Println() fmt.Println("Elements after sorting in lexicographical order.") // printing the elements after sorting them in lexicographical order for i := 0; i < 5; i++ { fmt.Print(stringSlice[i], " ") } fmt.Println() }
Output
Program to sort the element in lexicographical order using sort package. Elements before sorting in lexicographical order. Tutorial Point Java C++ Golang Elements after sorting in lexicographical order. C++ Golang Java Point Tutorial
Conclusion
These are the two ways to sort the elements in lexicographical order in Golang. The second way is much better in terms of modularity and code reusability as we can call that function anywhere in the project. To learn more about Golang you can explore these tutorials.