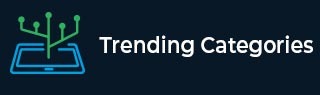
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to show Matplotlib in Flask?
To show a plot in Flask, we can take the following steps−
- Make a small application.
- To run Flask application, go to the current directory.
- $ export FLASK_APP=file.py
- $ flask run
- Open the browser, hit url:http://127.0.0.1:5000/print-plot/
- To plot the figure, we can create data points for x and y using random.
- Plot data points, x and y, on the created axis.
- Write a figure into png figure format.
- Retrieve the entire contents of the BytesIO object.
Example
import io from flask import Response from matplotlib.backends.backend_agg import FigureCanvasAgg as FigureCanvas from matplotlib.figure import Figure from flask import Flask import numpy as np plt.rcParams["figure.figsize"] = [7.50, 3.50] plt.rcParams["figure.autolayout"] = True app = Flask(__name__) @app.route('/print-plot') def plot_png(): fig = Figure() axis = fig.add_subplot(1, 1, 1) xs = np.random.rand(100) ys = np.random.rand(100) axis.plot(xs, ys) output = io.BytesIO() FigureCanvas(fig).print_png(output) return Response(output.getvalue(), mimetype='image/png')
Output
Advertisements