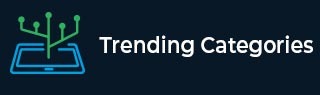
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to setup Video.js with ReactJS?
Video.js is a simple, straightforward, and easy-to-use modern video player which lets supports a video variety of video playback formats like mp4, FLV, etc. as well as modern formats like video playback formats like YouTube, Vimeo, flash, etc.
The best thing about video.js is that is very easy to import and use in our project. In this article, we're going to see how to use the video.js library in our React project with the help of a few examples.
How to setup Video.js with ReactJS?
For using video.js in our project with react.js, we first need to create a react component in our project and then we will use that component at various places in our project.
In this section of the tutorial, we're going to see how to create a functional component and how to use it in our project. Then, in the later half of the tutorial, we'll try to understand how to make a video-js class component and its usage within our project.
Functional Component (useEffect)
Let's start by creating a video-js functional component using the useEffect React hook, which will return a video element with the “video-js” class, and later we can reuse it in different parts of our project.
Consider the following react.js example to create a functional component for videojs.
import React from 'react'; import videojs from 'video.js'; import 'video-js.css'; export const VideoJS = (props) => { const videoReference = React.useRef(null); const playerReference = React.useRef(null); const {options, onReady} = props; React.useEffect(() => { // Initializing video.js player if (!playerReference.current) { const videoElement = videoReference.current; if (!videoElement) return; const player = playerReference.current = videojs(videoElement, options, () => { videojs.log('Video player is ready'); onReady && onReady(player); }); } }, [options, videoReference]); // Destroy video.js player on component unmount React.useEffect(() => { const player = playerReference.current; return () => { if (player) { player.dispose(); playerReference.current = null; } }; }, [playerReference]); // wrap player with data-vjs-player` attribute // so no additional wrapper are created in the DOM return ( <div data-vjs-player> <video ref={videoRef} className='video-js vjs-big-playcentered'/> </div> ); } export default VideoJS;
In the above piece of code, we've done the following −
First, we've imported react-js, video-js and video-js-css.
Then, we've created a functional Component by the name of “VideoJS” which is returning a video player or <video> element with className as 'video-js'
The functional component initialized the video player if there is no current video reference available and logs “Video Playe is ready”.
It also destroyed the player reference when the functional component is unmounted
Now that we've created a Video.js functional component, let's see how we can use this component in our project.
import React from 'react'; // importing the VideoJS Functional component import VideoJS from './VideoJS' const App = () => { const playerReference = React.useRef(null); // setting the video-js option for the player const videoJsOptions = { autoplay: true, controls: true, responsive: true, fluid: true, sources: [{ src: 'https://www.tutorialspoint.com/videos/sample480.mp4', type: 'video/mp4' }] }; const playerReady = (player) => { playerReference.current = player; // handling video player player.on('waiting', () => { videojs.log('Video Player is waiting'); }); player.on('dispose', () => { videojs.log('Video player will dispose'); }); }; return ( <> <VideoJS options={videoJsOptions} onReady={playerReady} /> </> ); }
In the above example, first we've imported the VideoJS functional component that we created earlier in the article. We've initialized the VideoJs Component below at the bottom of the page with videoJsOptions and some onReady functions.
videoJsOptions have also been defined, where we're setting some attributes for the video player like controls, autoplay, responsive, fluid, etc along with the sources video array. The sources array contains the path to the video files that we want to add with their mine type.
“playerReady” handles all the functions and handlers that will be executed when our video player is in 'waiting' state or is being disposed off.
Executing the above code in your React project is going to make an mp4 video player in the web browser. Now that we've understood the functional components, let's have a look at how to create class components.
Class Component
In the class component, we're again going to initialize a video player reference using video-js. This time we're going to use the React Life Cycle Hooks like 'componentDidMount' and 'componentWillUnmount' to handle various stages of our video player.
Consider the code example below to create a video-js class component that returns a video-js player.
import React from 'react'; import videojs from 'video.js'; import 'video.js/dist/video-js.css'; export default class VideoJSPlayer extends React.Component { // Initialize video.js player instance componentDidMount() { this.player = videojs(this.videoNode, this.props, () => { videojs.log('video player ready', this); }); } // Destroy video.js player reference componentWillUnmount() { if (this.player) { this.player.dispose(); } } // wrap player with data-vjs-player` attribute // so no additional wrapper are created in the DOM render() { return ( <div data-vjs-player> <video ref={node => this.videoNode = node} className="video-js"></video> </div> ); } }
In the above class component example, we've done the following −
First, we've imported reactjs, video-js and video-js css.
Next, we've created a class component named VideoJSPlayer which returns a video player created with className as “video-js”
We've initialized the video player when the component mounts using the react componentDidMount() hook and the video player reference is also being destroyed when the component unmounts using componentWillUnmount() hook.
Now that we've created a Video.js class component, let's see how we can use this component in our project.
Consider the code below to use the VideoJSPlayer Class Component −
import React from 'react'; // importing the VideoJS Functional component import VideoJSPlayer from './VideoJS' const App = () => { // setting the video-js option for the player const videoJsOptions = { autoplay: true, controls: true, responsive: true, fluid: true, sources: [{ src: 'https://www.tutorialspoint.com/videos/sample480.mp4', type: 'video/mp4' }] }; return <VideoJSPlayer {...videoJsOptions} /> }
In the above code snippet, we've first imported the “VideoJSPlayer” Class component which we've created in the earlier part of the article. Next, we've set some options for our video player like autoplay, controls, responsive and fluid along with the sources array. The sources array contains the path and type of all the video files that we need to create a video player for. Later at the bottom of the code excerpt, we've returned the VideoJSPlayer along with the videoJSOptions.
Executing the above code is going to create a video player for an mp4 file in the web browser but this time we've used a class component instead of a functional component.
Conclusion
In this tutorial, we understood how to set up video.js with react.js framework in our project. We had a look at the two methods which can be used to create a video.js component in react.js. Further, we created the video.js functional component and understood its usage with the help of an example. And in the second part of the tutorial, we created a class component and again saw how to use the same to create a video player in react.js.